python代码,利用Minimax算法的思想实现井字棋游戏
时间: 2024-11-07 21:25:01 浏览: 19
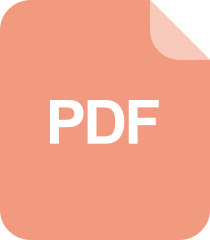
python使用minimax算法实现五子棋
在Python中,我们可以使用Minimax算法来实现井字棋(Tic Tac Toe)游戏。这是一种博弈树搜索算法,常用于两人对弈的游戏中,比如国际象棋。Minimax算法的目标是最大化玩家A的优势,同时最小化对手B的优势。以下是简单的实现步骤:
```python
class TicTacToe:
def __init__(self):
self.board = [' ' for _ in range(9)]
self.current_player = 'X'
def is_valid_move(self, move):
return self.board[move] == ' '
def make_move(self, move):
if not self.is_valid_move(move):
print("Invalid move")
return False
self.board[move] = self.current_player
self.current_player = 'O' if self.current_player == 'X' else 'X'
return True
def get_board_state(self):
return ''.join(self.board)
def minimax(board, depth, maximizing_player):
# Base case: Game over or maximum depth reached
if check_win(board) or len(get_valid_moves(board)) == 0 or depth == 0:
return evaluate_board_state(board), depth
if maximizing_player:
best_score = float('-inf')
for move in get_valid_moves(board):
new_board = apply_move(board, move)
score, _ = minimax(new_board, depth - 1, False)
best_score = max(score, best_score)
return best_score, depth
else:
worst_score = float('inf')
for move in get_valid_moves(board):
new_board = apply_move(board, move)
score, _ = minimax(new_board, depth - 1, True)
worst_score = min(score, worst_score)
return worst_score, depth
# Helper functions to check win and generate valid moves
def check_win(board):
# Add your implementation here...
def get_valid_moves(board):
# Add your implementation here...
# Main game loop
game = TicTacToe()
while True:
move = input("Player X, enter your move (1-9): ")
if game.make_move(int(move)):
current_score, _ = minimax(game.get_board_state(), 5, False) # Minimax with a depth of 5
print(f"Current score: {current_score}")
```
在这个例子中,`minimax`函数会递归地模拟所有可能的后续步骤,对于每个玩家,最大化他们的得分(如果是最大化玩家)或最小化对方的得分(如果是最小化玩家)。当你在游戏中移动时,它还会计算当前的最佳策略。
阅读全文
相关推荐
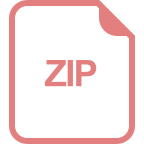
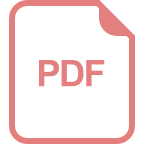
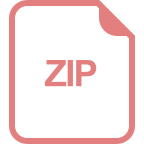
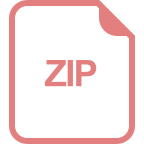
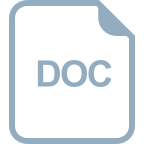
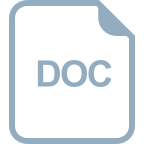
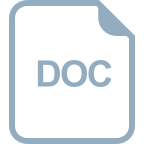
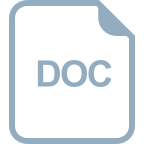


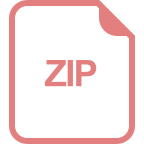
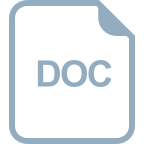
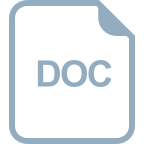
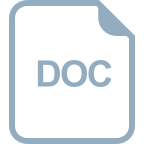
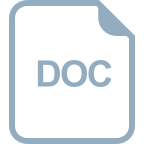
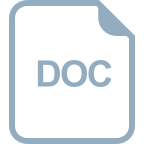
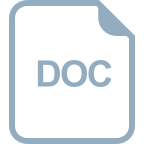
