用c语言写一个背单词程序
时间: 2024-06-14 07:04:55 浏览: 114
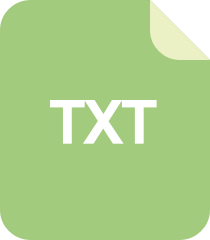
c语言背单词程序
以下是一个用C语言编写的简单背单词程序的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <time.h>
#define MAX_NAME_LENGTH 50
#define MAX_WORD_LENGTH 100
#define MAX_WORDS 100
typedef struct {
char chinese[MAX_WORD_LENGTH];
char english[MAX_WORD_LENGTH];
} Word;
void readWordsFromFile(Word words[], int *numWords) {
FILE *file = fopen("words.txt", "r");
if (file == NULL) {
printf("无法打开文件!\n");
exit(1);
}
*numWords = 0;
while (fscanf(file, "%s %s", words[*numWords].chinese, words[*numWords].english) == 2) {
(*numWords)++;
}
fclose(file);
}
void saveRecordToFile(char name[MAX_NAME_LENGTH], int numCorrect) {
FILE *file = fopen("record.txt", "a");
if (file == NULL) {
printf("无法打开文件!\n");
exit(1);
}
fprintf(file, "%s %d\n", name, numCorrect);
fclose(file);
}
void showWord(Word word) {
printf("中文:%s\n", word.chinese);
printf("英文:");
}
int main() {
char name[MAX_NAME_LENGTH];
int numWords;
Word words[MAX_WORDS];
int numCorrect = 0;
printf("请输入您的姓名:");
scanf("%s", name);
printf("请输入需要背的单词个数:");
scanf("%d", &numWords);
readWordsFromFile(words, &numWords);
srand(time(NULL));
for (int i = 0; i < numWords; i++) {
int randomIndex = rand() % numWords;
Word word = words[randomIndex];
showWord(word);
char answer[MAX_WORD_LENGTH];
scanf("%s", answer);
if (strcmp(answer, word.english) == 0) {
printf("回答正确!\n");
numCorrect++;
} else {
printf("回答错误!正确答案是:%s\n", word.english);
}
}
printf("您一共回答正确了%d个单词。\n", numCorrect);
saveRecordToFile(name, numCorrect);
return 0;
}
```
阅读全文
相关推荐
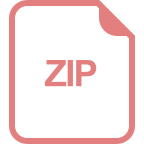
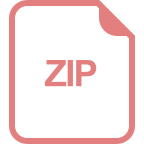
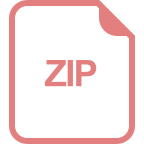
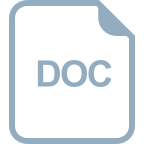
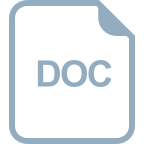



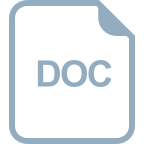
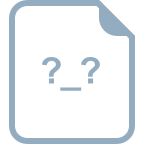
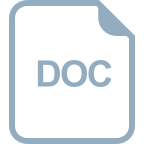
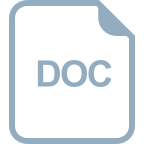
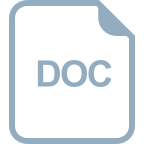