python使用Cryptodome库实现sm4_FPE_FF3的详细代码实现,Cryptodome提供了sm4基本的算法实现,非常详细
时间: 2024-10-25 14:06:54 浏览: 72
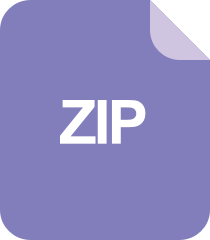
python-fpe:FPE-在Python中使用FF3进行格式保留加密

在Python中,使用`pycryptodome`库来实现SM4(FPE,Format-Preserving Encryption)的FF3模式(Fast Format Preserving Encryption)需要先安装`pycryptodome`库,如果你还没有安装,可以使用以下命令:
```bash
pip install pycryptodome
```
接下来是一个使用`Crypto.Cipher.SM4`模块实现FF3模式加密的简单示例:
```python
from Crypto.Cipher import SM4
from Crypto.Util.Padding import pad, unpad
from binascii import unhexlify, hexlify
# SM4 key (16 bytes for SM4)
key = b"your_secret_key_16_bytes"
def sm4_ff3_encrypt(plaintext, mode='CFB', iv=None):
cipher = SM4.SM4(key)
# FF3模式通常需要明文按照特定格式填充
if mode == 'CFB':
padding_size = 16 - (len(plaintext) % 16)
padded_plaintext = pad(plaintext.encode(), padding_size)
ciphertext = cipher.encrypt(padded_plaintext, iv=iv)
return hexlify(ciphertext).decode()
def sm4_ff3_decrypt(ciphertext, original_plaintext_len, mode='CFB', iv=None):
key = b"your_secret_key_16_bytes"
cipher = SM4.SM4(key)
encrypted_data = unhexlify(ciphertext)
padded_ciphertext = cipher.decrypt(encrypted_data, iv=iv)
unpadded_ciphertext = unpad(padded_ciphertext, 16)
# 如果有模式信息,可以根据原始长度还原填充
if len(original_plaintext_len) > 0:
return unpadded_ciphertext[:original_plaintext_len]
else:
return unpadded_ciphertext
# 示例
plaintext = "Hello, World!"
ciphertext = sm4_ff3_encrypt(plaintext, iv=b"your_initialization_vector")
print("Ciphertext:", ciphertext)
# 解密
original_length = len(plaintext.encode())
decrypted_text = sm4_ff3_decrypt(ciphertext, original_length)
print("Decrypted Text:", decrypted_text)
```
注意,这只是一个基本示例,实际应用中你可能需要根据具体需求调整填充策略和处理模式信息的方式。
阅读全文
相关推荐
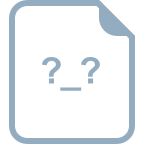




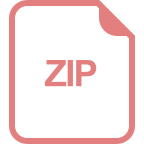
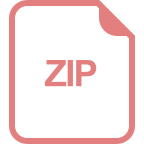
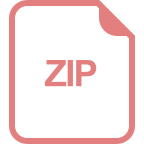
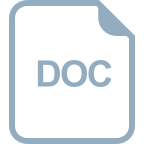
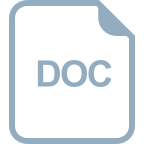
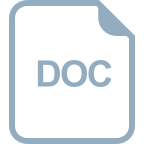
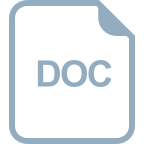
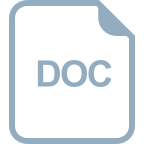

