python获取本机的IP地址,非127开头
时间: 2024-10-22 14:11:06 浏览: 24
在Python中,你可以通过内置模块`socket`来获取本机的IP地址,尤其是公网IP地址。下面是一个简单的示例:
```python
import socket
def get_public_ip():
try:
# 连接Google公共DNS服务器
s = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
s.connect(('8.8.8.8', 80))
# 获取IP地址,第一个元素就是公网IP
ip_address = s.getsockname()[0]
s.close()
return ip_address
except Exception as e:
print(f"无法获取IP: {e}")
return None
public_ip = get_public_ip()
if public_ip is not None and not public_ip.startswith('127'):
print(f"Your public IP address is: {public_ip}")
else:
print("Public IP address cannot be retrieved or is a loopback address.")
相关问题
帮我写个python脚本,根据IP和子网掩码获取所有包含的IP
好的,下面是一个根据IP和子网掩码计算所有包含的IP地址的简单脚本:
```
import socket
import struct
def get_ip_range(ip, subnet_mask):
ip_int = struct.unpack("!I", socket.inet_aton(ip))[0]
subnet_int = struct.unpack("!I", socket.inet_aton(subnet_mask))[0]
network_int = ip_int & subnet_int
broadcast_int = network_int | ~subnet_mask
network = socket.inet_ntoa(struct.pack("!I", network_int))
broadcast = socket.inet_ntoa(struct.pack("!I", broadcast_int))
return network, broadcast
ip = '192.168.1.100'
subnet_mask = '255.255.255.0'
network, broadcast = get_ip_range(ip, subnet_mask)
print('Network:', network)
print('Broadcast:', broadcast)
ips = []
for i in range(0, 255):
ips.append(socket.inet_ntoa(struct.pack('!I', struct.unpack('!I', socket.inet_aton(network))[0] + i)))
print('IPs:', ips)
```
使用方法:
- 在程序的开头,定义了两个变量 `ip` 和 `subnet_mask`,请根据需要替换为你想要查询的IP地址和子网掩码。
- 运行该脚本,程序会先输出网络地址和广播地址,再输出所有的可用IP地址。
希望对您有帮助!
配置文件为#global file.charset=UTF-8(none BOM) #服务器IP地址 machine.ip=10.19.133.38 #根秘钥生成部件(partB),随机生成,使用base64编码 encrype.part=LF2Ko+wB3uvsIW5ih3+GqQ==,python2,已知配置文件路径/opt/hikvision/web/opsMgrCenter/conf和名称config.properties,想要获取配置文件中machine.ip=127.0.0.1中的127.0.0.1
在这个配置文件中,第一行以`#`开头,这意味着它是一个注释行。而`file.charset=UTF-8(none BOM)`这一行则不是一个有效的段落头部,因此它会被忽略掉。
现在,你需要使用Python2中的ConfigParser模块来读取配置文件并获取指定的值。可以使用下面的代码:
```python
import ConfigParser
# 创建ConfigParser对象
config = ConfigParser.RawConfigParser()
# 读取配置文件
config.read('/opt/hikvision/web/opsMgrCenter/conf/config.properties')
# 获取指定section下的指定key对应的value
ip = config.get('default', 'machine.ip')
print(ip)
```
在这个代码中,我们使用了`RawConfigParser`类来创建ConfigParser对象,因为它可以在不区分大小写的情况下读取配置文件。我们还指定了配置文件的完整路径和名称`/opt/hikvision/web/opsMgrCenter/conf/config.properties`。
最后,我们使用`get`方法来获取`machine.ip`键对应的值,该键在`default`段落下。注意,在配置文件中,`machine.ip`的值是`10.19.133.38`而不是`127.0.0.1`,因此你可能需要修改该值来获取正确的结果。
阅读全文
相关推荐
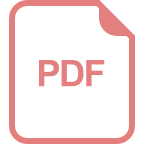
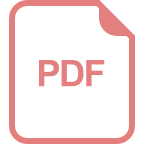
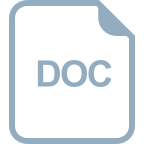
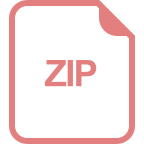
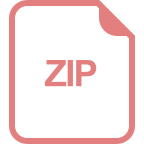
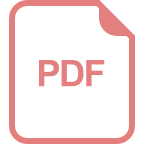
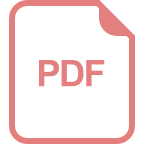
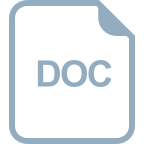
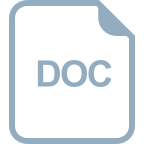
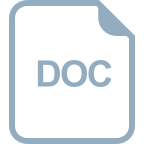
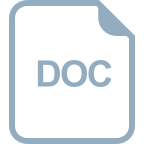
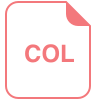
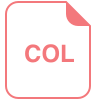
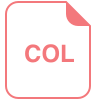
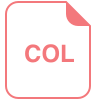
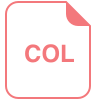
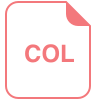