写一个python射线追踪代码
时间: 2023-02-23 12:29:26 浏览: 343
射线追踪是一种用于模拟光线在三维空间中的行为的计算机图形学技术。下面是一个简单的 Python 射线追踪代码示例:
```
from math import sqrt
class Ray:
def __init__(self, origin, direction):
self.origin = origin
self.direction = direction
def point_at_parameter(self, t):
return self.origin + self.direction * t
class Sphere:
def __init__(self, center, radius):
self.center = center
self.radius = radius
def hit(self, ray, t_min, t_max):
oc = ray.origin - self.center
a = ray.direction.dot(ray.direction)
b = 2.0 * oc.dot(ray.direction)
c = oc.dot(oc) - self.radius * self.radius
discriminant = b * b - 4 * a * c
if discriminant > 0:
root = sqrt(discriminant)
temp = (-b - root) / (2.0 * a)
if t_min < temp < t_max:
return temp
temp = (-b + root) / (2.0 * a)
if t_min < temp < t_max:
return temp
return None
def color(ray, sphere):
hit_point = None
t = sphere.hit(ray, 0.001, float('inf'))
if t:
hit_point = ray.point_at_parameter(t)
normal = (hit_point - sphere.center) / sphere.radius
return 0.5 * (normal + 1)
else:
return None
```
这是一个非常简单的射线追踪代码,仅涉及到球体与光线的交点求解。在实际使用中,您可能需要添加更多复杂的几何体和光线效果。
阅读全文
相关推荐
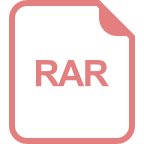
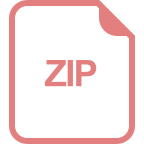


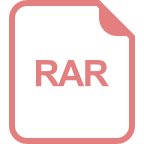
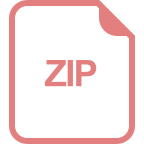
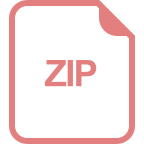
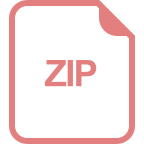
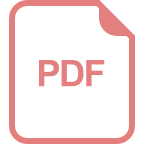
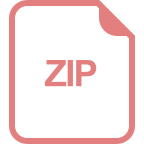
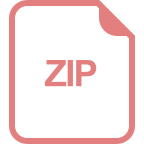
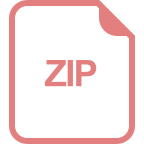
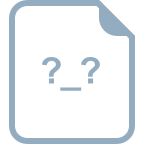
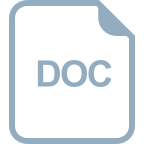
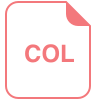

