def CaptureImages(window_name,student_id): capture_pic_num = 100 folder_path = f"C:/Users/DELL/PycharmProjects/pythonProject/{student_id}" os.makedirs(folder_path, exist_ok=True) cv2.namedWindow(window_name) cap = cv2.VideoCapture(0) classifier = cv2.CascadeClassifier("haarcascade_frontalface_alt2.xml") color = (0, 255, 0) num = 0 while cap.isOpened(): ret, frame = cap.read() if not ret: break gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY) faces = classifier.detectMultiScale(gray, scaleFactor=1.2, minNeighbors=3, minSize=(32, 32)) if len(faces) > 0: for (x, y, w, h) in faces: img_path = f"{folder_path}/{num}.jpg" image = frame[y - 10:y + h + 10, x - 10:x + w + 10] cv2.imwrite(img_path, image) num += 1 cv2.rectangle(frame, (x - 10, y - 10), (x + w + 10, y + h + 10), color, 2) font = cv2.FONT_HERSHEY_SIMPLEX cv2.putText(frame, f'num:{num}', (x + 30, y + 30), font, 1, (255, 0, 255), 4) if num >= capture_pic_num: break cv2.imshow(window_name, frame) if cv2.waitKey(30) & 0xFF == ord('q'): break cap.release() cv2.destroyAllWindows()怎么将这个功能与一个qt界面的按钮相连接
时间: 2024-02-14 14:26:00 浏览: 100
要将这个功能与一个Qt界面的按钮相连接,你需要按照以下步骤进行操作:
1. 导入必要的模块和库:
```python
import cv2
import os
from PyQt5.QtWidgets import QApplication, QMainWindow, QPushButton, QVBoxLayout, QWidget
from PyQt5.QtCore import Qt, QThread
from PyQt5.QtGui import QImage, QPixmap
```
2. 创建一个 Qt 主窗口类(例如 `MainWindow`):
```python
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle("Capture Images")
self.setFixedSize(300, 200)
# 创建按钮并连接到槽函数
self.capture_btn = QPushButton("Capture", self)
self.capture_btn.clicked.connect(self.start_capture)
# 创建布局,将按钮放入布局中,并设置布局为窗口的中心布局
layout = QVBoxLayout()
layout.addWidget(self.capture_btn)
central_widget = QWidget()
central_widget.setLayout(layout)
self.setCentralWidget(central_widget)
# 按钮点击事件的槽函数
def start_capture(self):
# 在这里调用 CaptureImages 函数进行图像捕获
CaptureImages("Capture Images", "student_id")
```
3. 创建一个线程类(例如 `CaptureThread`),用于在后台执行图像捕获操作:
```python
class CaptureThread(QThread):
def run(self):
CaptureImages("Capture Images", "student_id")
```
4. 在主程序中创建 Qt 应用程序并启动主窗口:
```python
if __name__ == "__main__":
app = QApplication([])
main_window = MainWindow()
main_window.show()
app.exec_()
```
这样,当你点击按钮时,它将调用 `start_capture` 槽函数,然后在后台启动一个线程来执行 `CaptureImages` 函数进行图像捕获操作。请注意,你需要将 `student_id` 更改为实际的学生ID。
希望这可以帮助到你!如果你有任何其他问题,请随时问我。
阅读全文
相关推荐
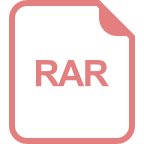
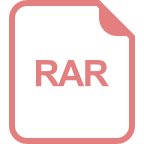
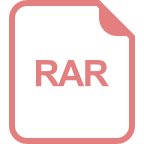
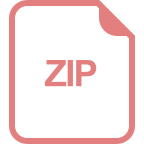
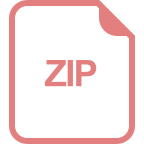
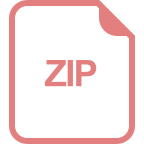
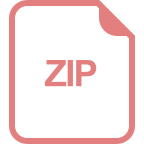
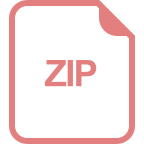
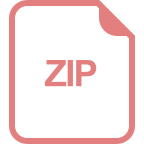
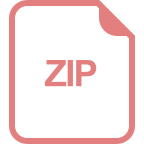
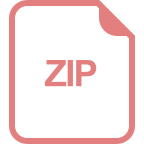
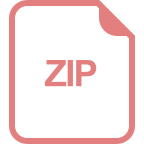
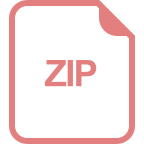
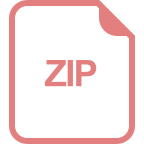
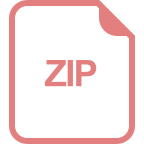
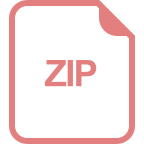
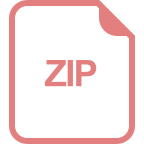
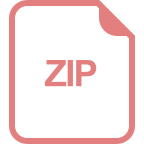
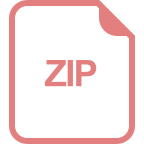