用python写一个贪吃蛇
时间: 2023-07-01 15:16:05 浏览: 40
好的,以下是一个简单的贪吃蛇游戏的 Python 代码:
```
import pygame
import random
# 初始化 pygame
pygame.init()
# 创建游戏窗口
window_width = 800
window_height = 600
window = pygame.display.set_mode((window_width, window_height))
pygame.display.set_caption("贪吃蛇")
# 定义颜色
white = (255, 255, 255)
black = (0, 0, 0)
red = (255, 0, 0)
green = (0, 255, 0)
# 定义贪吃蛇类
class Snake:
def __init__(self):
self.x = window_width // 2
self.y = window_height // 2
self.direction = "right"
self.length = 1
self.body = [(self.x, self.y)]
def move(self):
if self.direction == "right":
self.x += 10
elif self.direction == "left":
self.x -= 10
elif self.direction == "up":
self.y -= 10
elif self.direction == "down":
self.y += 10
self.body.append((self.x, self.y))
if len(self.body) > self.length:
del self.body[0]
def draw(self):
for x, y in self.body:
pygame.draw.rect(window, green, [x, y, 10, 10])
# 定义食物类
class Food:
def __init__(self):
self.x = random.randrange(0, window_width-10, 10)
self.y = random.randrange(0, window_height-10, 10)
def draw(self):
pygame.draw.rect(window, red, [self.x, self.y, 10, 10])
# 创建贪吃蛇和食物对象
snake = Snake()
food = Food()
# 定义游戏循环
clock = pygame.time.Clock()
game_over = False
while not game_over:
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_over = True
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_RIGHT and snake.direction != "left":
snake.direction = "right"
elif event.key == pygame.K_LEFT and snake.direction != "right":
snake.direction = "left"
elif event.key == pygame.K_UP and snake.direction != "down":
snake.direction = "up"
elif event.key == pygame.K_DOWN and snake.direction != "up":
snake.direction = "down"
# 移动贪吃蛇和判断是否吃到食物
snake.move()
if snake.body[-1][0] == food.x and snake.body[-1][1] == food.y:
snake.length += 1
food = Food()
# 判断贪吃蛇是否撞到边界或自己
if snake.body[-1][0] < 0 or snake.body[-1][0] >= window_width or snake.body[-1][1] < 0 or snake.body[-1][1] >= window_height:
game_over = True
for i in range(len(snake.body)-1):
if snake.body[-1][0] == snake.body[i][0] and snake.body[-1][1] == snake.body[i][1]:
game_over = True
# 绘制游戏画面
window.fill(white)
snake.draw()
food.draw()
pygame.display.update()
# 控制游戏帧率
clock.tick(10)
# 退出游戏
pygame.quit()
quit()
```
运行上述代码会打开一个窗口,可以通过方向键控制贪吃蛇的移动,当贪吃蛇吃到食物时,长度会增加,当贪吃蛇撞到边界或自己时,游戏结束。
相关推荐
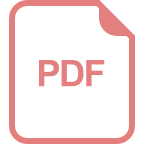
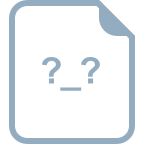

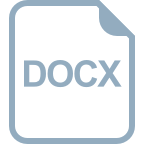
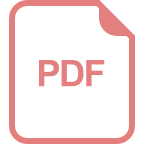
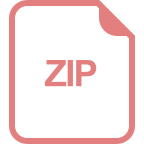
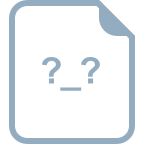
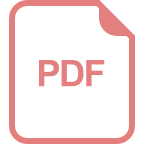
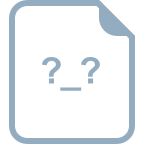
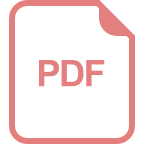
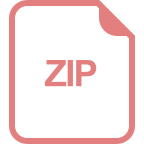