#include <iostream> #include <algorithm> #include <vector> using namespace std; void printVector(vector<int>& v) { for (int i = 0; i < v.size(); i++) { cout << v[i] << " "; } cout << endl; } void permutationSelectionSort(vector<int>& nums, int heapSize) { int n = nums.size(); int start = 0; while (start < n) { int maxIndex = start; for (int i = start + 1; i < min(start + heapSize, n); i++) { if (nums[i] > nums[maxIndex]) { maxIndex = i; } } // 交换最大值和当前堆的第一个元素 swap(nums[start], nums[maxIndex]); // 对当前堆进行选择排序,将其排序为从小到大的顺序 for (int i = start + 1; i < min(start + heapSize, n); i++) { int j = i; while (j > start && nums[j] < nums[j - 1]) { swap(nums[j], nums[j - 1]); j--; } } start += heapSize; } } int main() { vector<int> nums = {50, 49, 35, 45, 30, 25, 15, 60, 27}; int heapSize = 3; permutationSelectionSort(nums, heapSize); for (int i = 0; i < nums.size(); i += heapSize) { printVector(vector<int>(nums.begin() + i, nums.begin() + min(i + heapSize, (int)nums.size()))); } return 0; }
时间: 2024-01-15 15:02:49 浏览: 34
在您提供的代码中,函数`printVector`和`permutationSelectionSort`的参数`vector<int>&`使用了非常量引用,但是在函数调用时并没有传入左值,导致出现了错误。可以通过将函数参数修改为`const vector<int>&`来解决这个问题,即:
```
void printVector(const vector<int>& v) {
for (int i = 0; i < v.size(); i++) {
cout << v[i] << " ";
}
cout << endl;
}
void permutationSelectionSort(vector<int>& nums, int heapSize) {
int n = nums.size();
int start = 0;
while (start < n) {
int maxIndex = start;
for (int i = start + 1; i < min(start + heapSize, n); i++) {
if (nums[i] > nums[maxIndex]) {
maxIndex = i;
}
}
// 交换最大值和当前堆的第一个元素
swap(nums[start], nums[maxIndex]);
// 对当前堆进行选择排序,将其排序为从小到大的顺序
for (int i = start + 1; i < min(start + heapSize, n); i++) {
int j = i;
while (j > start && nums[j] < nums[j - 1]) {
swap(nums[j], nums[j - 1]);
j--;
}
}
start += heapSize;
}
}
```
修改后,以上代码应该就可以正常运行了。
相关推荐
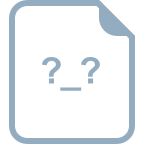







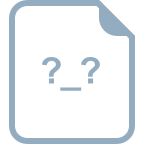
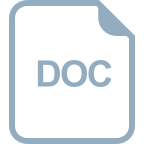
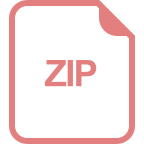
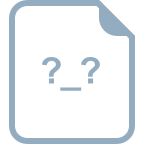
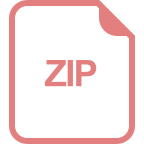
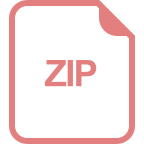