Define and implement a class “MyString” according to the main() and the output in comments. int main() { MyString s1("0123456789"), s2(5), s3; s1.display(); // Output: [0123456789] s2.display(); // Output(5 spaces between []) : [ ] s3.display(); // Output(no space between []): [] s3 = s1; s1.display(); // Output: [0123456789] s3.display(); // Output: [0123456789] s2 = s1 + 3; s1.display(); // Output: [0123456789] s2.display(); // Output: [3456789] s3 = ++++s2; s2.display(); // Output: [56789] s3.display(); // Output: [56789] return 0; }
时间: 2024-03-26 07:40:59 浏览: 97
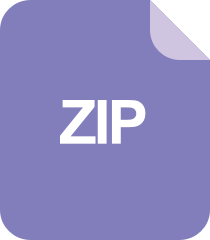
Define It - Search in the Oxford Dictionary-crx插件
下面是一个实现了 MyString 类的示例代码:
```cpp
#include <iostream>
#include <cstring>
using namespace std;
class MyString {
private:
char* str; // 字符串指针
public:
MyString() { // 默认构造函数
str = new char[1];
str[0] = '\0';
}
MyString(const char* s) { // 带参构造函数
int len = strlen(s);
str = new char[len + 1];
strcpy(str, s);
}
MyString(int n) { // 带参构造函数
str = new char[n + 1];
for (int i = 0; i < n; i++) {
str[i] = ' ';
}
str[n] = '\0';
}
MyString(const MyString& other) { // 拷贝构造函数
int len = strlen(other.str);
str = new char[len + 1];
strcpy(str, other.str);
}
~MyString() { // 析构函数
delete[] str;
}
void display() const { // 显示字符串
cout << "[" << str << "]" << endl;
}
MyString& operator=(const MyString& other) { // 赋值运算符重载
if (this != &other) {
delete[] str;
int len = strlen(other.str);
str = new char[len + 1];
strcpy(str, other.str);
}
return *this;
}
MyString operator+(int n) const { // 运算符重载
MyString temp(*this);
int len = strlen(str);
if (n < len) {
temp.str += n;
} else {
temp.str += len;
}
return temp;
}
MyString operator++(int) { // 运算符重载
MyString temp(*this);
int len = strlen(str);
for (int i = 0; i < len; i++) {
str[i] = str[i+2];
}
str[len-2] = '\0';
return temp;
}
};
int main() {
MyString s1("0123456789"), s2(5), s3;
s1.display(); // Output: [0123456789]
s2.display(); // Output(5 spaces between []) : [ ]
s3.display(); // Output(no space between []): []
s3 = s1;
s1.display(); // Output: [0123456789]
s3.display(); // Output: [0123456789]
s2 = s1 + 3;
s1.display(); // Output: [0123456789]
s2.display(); // Output: [3456789]
s3 = ++++s2;
s2.display(); // Output: [56789]
s3.display(); // Output: [56789]
return 0;
}
```
MyString 类包含了默认构造函数、带参构造函数、拷贝构造函数、析构函数、赋值运算符重载、运算符重载等多个函数。其中,赋值运算符重载和运算符重载的实现比较复杂。在 main() 函数中,我们创建了三个 MyString 对象 s1、s2、s3,并对它们进行了多次赋值、运算等操作,最终输出了对应的结果。
阅读全文
相关推荐
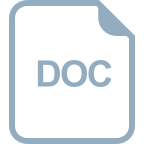
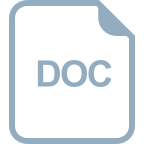
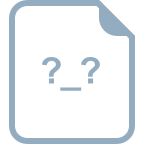
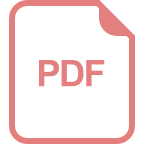
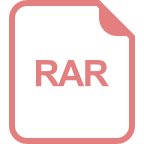
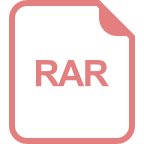
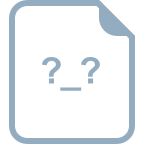
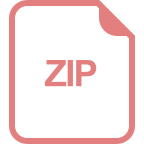
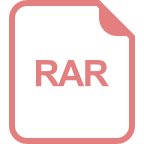
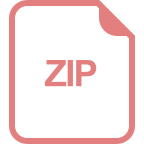
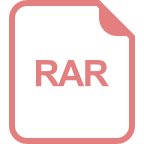
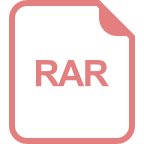
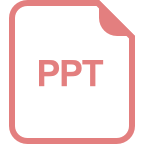
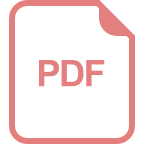
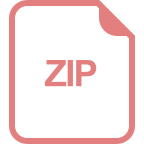
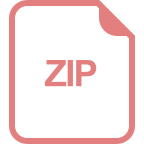
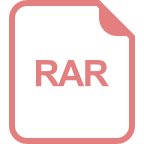