使用java用retrofit2实习高并发访问网站
时间: 2023-03-25 07:03:59 浏览: 207
可以使用 Retrofit2 的线程池来实现高并发访问网站,具体可以通过设置 OkHttpClient 的连接池大小、超时时间等参数来优化网络请求。同时,可以使用 RxJava 来实现异步请求和响应处理,从而提高并发能力。
相关问题
Retrofit在java中怎么使用
Retrofit是一个类型安全的HTTP客户端,用于Android和Java应用程序,它通过使用注解来描述HTTP请求,参数和响应体,从而将服务端API调用转化为Java接口的实现。下面将介绍如何在Java中使用Retrofit进行API调用:
1. **添加依赖**:在项目中添加Retrofit的依赖项,如果你使用的是Gradle构建工具,可以在build.gradle文件中添加如下依赖:
```java
implementation 'com.squareup.retrofit2:retrofit:2.9.0'
```
2. **创建Retrofit实例**:创建一个Retrofit实例需要提供两个主要的东西:一个基础URL和一个OkHttpClient实例。基础URL是API的基础路径,OkHttpClient用于配置网络请求的属性,如超时时间等。
```java
Retrofit retrofit = new Retrofit.Builder()
.baseUrl("https://api.example.com/")
.client(new OkHttpClient())
.addConverterFactory(GsonConverterFactory.create())
.build();
```
3. **定义服务接口**:创建一个接口作为API的抽象定义,通过在接口方法上添加注解来描述HTTP请求的细节,例如@GET、@POST等。
```java
public interface ApiService {
@GET("user/{id}")
Call<User> getUser(@Path("id") int userId);
}
```
4. **生成API对象**:利用之前创建的Retrofit实例来生成API对象。
```java
ApiService apiService = retrofit.create(ApiService.class);
```
5. **发起API调用**:现在可以通过API对象发起同步或异步的API调用了。
```java
// 同步调用
User user = apiService.getUser(1).execute().body();
// 异步调用
Call<User> call = apiService.getUser(1);
call.enqueue(new Callback<User>() {
@Override
public void onResponse(Call<User> call, Response<User> response) {
if (response.isSuccessful()) {
User user = response.body();
// 处理用户数据
} else {
// 处理错误情况
}
}
@Override
public void onFailure(Call<User> call, Throwable t) {
// 处理失败情况
}
});
```
通过以上步骤,你就可以在Java项目中使用Retrofit进行高效的API调用了。记得替换示例中的URL和API接口为你实际项目中的地址和接口。
Springboot使用retrofit2
Spring Boot与Retrofit2可以很好地结合使用,以方便地进行网络请求。下面是使用Spring Boot和Retrofit2的基本步骤:
1. 添加依赖:在项目的pom.xml文件中添加以下依赖:
```xml
<dependencies>
<!-- Spring Boot Starter Web -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- Retrofit2 -->
<dependency>
<groupId>com.squareup.retrofit2</groupId>
<artifactId>retrofit</artifactId>
<version>2.9.0</version>
</dependency>
<!-- Retrofit2 Gson Converter -->
<dependency>
<groupId>com.squareup.retrofit2</groupId>
<artifactId>converter-gson</artifactId>
<version>2.9.0</version>
</dependency>
</dependencies>
```
2. 创建Retrofit实例:在Spring Boot的配置类中,使用`@Bean`注解创建一个Retrofit实例,并配置相关参数,如接口的基本URL、Gson转换器等。
```java
import okhttp3.OkHttpClient;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import retrofit2.Retrofit;
import retrofit2.converter.gson.GsonConverterFactory;
@Configuration
public class RetrofitConfig {
@Bean
public Retrofit retrofit() {
OkHttpClient client = new OkHttpClient.Builder()
// 可以添加自定义的OkHttpClient配置
.build();
return new Retrofit.Builder()
.baseUrl("http://api.example.com/") // 设置接口的基本URL
.client(client)
.addConverterFactory(GsonConverterFactory.create()) // 设置Gson转换器
.build();
}
}
```
3. 创建API接口:定义一个接口,用于描述请求的方法和参数。
```java
import retrofit2.Call;
import retrofit2.http.GET;
public interface ApiService {
@GET("users")
Call<List<User>> getUsers();
}
```
4. 使用Retrofit发起请求:在需要使用网络请求的地方,通过依赖注入的方式获取Retrofit实例,并调用接口方法发起请求。
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import retrofit2.Call;
import retrofit2.Response;
import java.io.IOException;
import java.util.List;
@Service
public class UserService {
@Autowired
private Retrofit retrofit;
public List<User> getUsers() throws IOException {
ApiService apiService = retrofit.create(ApiService.class);
Call<List<User>> call = apiService.getUsers();
Response<List<User>> response = call.execute();
if (response.isSuccessful()) {
return response.body();
} else {
throw new IOException("请求失败:" + response.code());
}
}
}
```
这样就可以在Spring Boot项目中使用Retrofit2进行网络请求了。注意要根据实际需求进行配置和调整,例如添加拦截器、设置超时时间等。
相关推荐
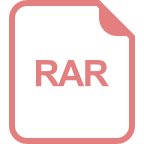













