springboot整合knife4j
时间: 2023-05-31 11:19:45 浏览: 105
### 回答1:
Knife4j是一个基于Swagger-Bootstrap-UI的开源项目,可以在Spring Boot中整合使用。
整合步骤如下:
1. 引入knife4j的依赖
2. 配置knife4j的相关参数
3. 在启动类上添加@EnableSwaggerBootstrapUi注解
4. 在需要使用knife4j的接口上添加@ApiOperation注解
5. 启动项目,访问http://{host}:{port}/swagger-ui.html即可查看接口文档
### 回答2:
Spring Boot是一种快速、便捷的应用开发框架,Knife4j是一种专业的Java接口文档生成工具,将它们两者结合起来可以提供一个更为便捷、高效的开发体验。下面是关于如何整合Spring Boot和Knife4j的详细过程:
1.引入依赖
在pom.xml中引入如下依赖:
```
<dependency>
<groupId>com.github.xiaoymin</groupId>
<artifactId>knife4j-spring-boot-starter</artifactId>
<version>3.0.2</version>
</dependency>
```
2. 应用配置
在application.yml文件中进行应用配置:
```
knife4j:
title: Demo API Docs
description: Demo API Description
version: "1.0"
license:
name: MIT
url: https://github.com/mit-license
contact:
name: Demo
url: https://github.com/demo
email: demo@qq.com
show: true
enable: true
scan-package: com.demo.controller
```
其中,title、description、version等属性可根据实际情况进行修改,scan-package则是指定需要扫描的API包名。
3. 编写API文档
在Controller类的方法上添加@Api注解,如下所示:
```
@RestController
@RequestMapping(value = "/user")
@Api(value = "用户相关接口", tags = {"用户相关接口"})
public class UserController {
@ApiOperation(value = "获取所有用户信息", notes = "获取所有用户信息")
@GetMapping(value = "/list")
public ResponseEntity<List<User>> list() {
// ...
}
}
```
其中,@Api注解指定API的名称和描述信息,@ApiOperation注解则指定每个API方法的名称和描述信息。
4. 启动应用
启动应用,浏览器访问http://localhost:8080/doc.html,即可查看生成的API文档。
总结
整合Spring Boot和Knife4j可以方便快捷地生成API文档,提高开发效率和开发者体验。通过上述步骤,可以快速上手并使用该工具。
### 回答3:
Spring Boot 是一种针对 Spring 框架的快速应用开发框架。而 Knife4j 是基于 Swagger 的文档生成工具,用来生成高质量的 API 文档,且支持在线调试和 Mock 数据。
对于 Spring Boot 的开发者来说,整合 Knife4j 可以帮助他们更加方便地生成 API 文档和在线调试,提高开发速度和效率。以下就是整合步骤:
1. 添加依赖和插件
在 pom.xml 文件中添加以下依赖和插件:
```
<!-- Swagger-UI -->
<dependency>
<groupId>com.github.xiaoymin</groupId>
<artifactId>swagger-bootstrap-ui</artifactId>
<version>1.9.6</version>
</dependency>
<!-- Swagger-Annotations -->
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger2</artifactId>
<version>2.9.2</version>
</dependency>
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger-ui</artifactId>
<version>2.9.2</version>
</dependency>
<!-- Knife4j -->
<dependency>
<groupId>com.github.xiaoymin</groupId>
<artifactId>knife4j-spring-boot-starter</artifactId>
<version>3.0.2</version>
</dependency>
<!-- 插件 -->
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
<!-- Swagger2Markup -->
<plugin>
<groupId>io.github.swagger2markup</groupId>
<artifactId>swagger2markup-maven-plugin</artifactId>
<version>${swagger2markup.version}</version>
<configuration>
<swaggerInput>${project.build.directory}/swagger/swagger.json</swaggerInput>
<outputDirAsPath>true</outputDirAsPath>
<outputDir>${project.build.directory}/docs</outputDir>
<config>
<swagger2markup.extensions.interceptors.paths>
<interceptor path="*.json"></interceptor>
</swagger2markup.extensions.interceptors.paths>
</config>
</configuration>
</plugin>
</plugins>
</build>
```
其中,Swagger-UI 是 Swagger 的界面展示工具,Knife4j 依赖于 Swagger-UI,因此需要同时引入 Swagger-UI 和 Swagger-Annotations。而 Swagger-Annotations 是用来标识接口的注解,通过这些注解生成接口文档。
2. 配置 Swagger
在 application.yml 或 application.properties 中添加以下配置:
```
## Swagger
swagger:
enabled: true
title: Knife4j整合Swagger2接口文档
description: Knife4j整合Swagger2,可以快速生成API文档
version: 2.0
contact:
name: FZF
url: http://fzf.blog.csdn.net/
basePackage: com.fzf.knife4j
host: localhost:8080
protocols: http,https
```
Property | Default | Comment
----------------|-----------------------|---------
`enabled` | true | 是否开启 Swagger
`title` | Application Name | Swagger 页面标题
`description` | EMPTY | Swagger 页面描述
`version` | 1.0 | API 版本号
`contact.name` | EMPTY | 联系人姓名
`contact.url` | EMPTY | 联系人 URL
`basePackage` | Application Package | 扫描的包路径
`host` | EMPTY | 访问地址
`protocols` | http | 协议,concatenated list of protocols (http, https, ws, wss)
3. 配置 Knife4j
在 application.yml 或 application.properties 中添加以下配置:
```
## Knife4j
knife4j:
enabled: true
title: Knife4j整合Swagger2接口文档
description: Knife4j整合Swagger2,可以快速生成API文档
version: 2.0
contact:
name: FZF
url: http://fzf.blog.csdn.net/
license:
name: Apache-2.0
url: http://www.apache.org/licenses/LICENSE-2.0
termsOfServiceUrl: https://github.com/fuzhengwei/swagger-bootstrap-ui/blob/master/LICENSE
basePackage: com.fzf.knife4j
versionGroup: v2
scanPackages: com.fzf.knife4j
host: localhost:8080
protocols: http,https
globalOperationParameters:
- name: authToken
description: token
modelRef: string
parameterType: header
required: false
```
Property | Default | Comment
--------------------------------|-------------------------|---------
`enabled` | true | 是否开启 Knife4j
`title` | Application Name | Knife4j 页面标题
`description` | EMPTY | Knife4j 页面描述
`version` | 1.0 | API 版本号
`contact.name` | EMPTY | 联系人姓名
`contact.url` | EMPTY | 联系人 URL
`license.name` | EMPTY | 许可证名称
`license.url` | EMPTY | 许可证 URL
`termsOfServiceUrl` | EMPTY | 服务条款 URL
`basePackage` | Application Package | 扫描的包路径
`versionGroup` | v1, v2, ... | API 版本组名
`scanPackages` | EMPTY | 扫描的包路径
`host` | EMPTY | 访问地址
`protocols` | http | 协议,concatenated list of protocols (http, https, ws, wss)
`globalOperationParameters` | EMPTY | 全局参数,例如鉴权 token 等
4. 创建 Swagger 配置类
在 Spring Boot 项目中创建一个 Swagger 配置类:
```
@Configuration
@EnableSwagger2
@Import(BeanValidatorPluginsConfiguration.class)
public class SwaggerConfiguration {
@Bean
public Docket api() {
return new Docket(DocumentationType.SWAGGER_2).apiInfo(apiInfo()).select()
.apis(RequestHandlerSelectors.basePackage("com.fzf.knife4j")).paths(PathSelectors.any()).build();
}
private ApiInfo apiInfo() {
return new ApiInfoBuilder().title("Knife4j整合Swagger2接口文档").description("Knife4j整合Swagger2,可以快速生成API文档")
.termsOfServiceUrl("https://github.com/fuzhengwei/swagger-bootstrap-ui").version("2.0").build();
}
}
```
其中,`@Configuration` 注解用于标识该类为 Spring 配置类;`@EnableSwagger2` 注解用于开启 Swagger2;`@Import(BeanValidatorPluginsConfiguration.class)` 注解用于启用 Hibernate Validator 注解,这是为了在 Swagger UI 中验证接口参数的有效性。`api()` 方法用于生成 Swagger Docket(API 文档),`apiInfo()` 方法用于指定 API 文档的基本信息,例如标题、描述、版本等。
5. 启动项目
在项目启动后,访问 http://localhost:8080/swagger-ui.html 可以看到 Swagger 的页面,访问 http://localhost:8080/doc.html 可以看到 Knife4j 的页面,访问 http://localhost:8080/docs/index.html 可以看到导出的文档。
总结:
通过以上步骤即可成功地将 Knife4j 整合到 Spring Boot 项目中,实现 API 文档的自动生成,以及在线调试和 Mock 数据功能。整合后的 API 文档可以更加规范、高效地管理和维护 API 文档,提高接口文档的可读性和可维护性,同时也提高了接口的开发效率和质量。
相关推荐
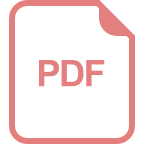














