java读取json文件内容
时间: 2023-09-14 09:06:32 浏览: 127
要在Java中读取JSON文件的内容,可以使用以下步骤:
1. 通过文件路径创建File对象:
```
File jsonFile = new File("path/to/json/file.json");
```
2. 使用Java IO流读取文件内容,并将其转换为字符串:
```
String jsonString = new String(Files.readAllBytes(jsonFile.toPath()));
```
3. 使用Java内置的JSON库将JSON字符串转换为JSON对象:
```
JSONObject jsonObject = new JSONObject(jsonString);
```
4. 现在你可以使用JSON对象中提供的方法来访问和操作JSON数据了。
完整的代码如下所示:
```java
import org.json.JSONObject;
import java.io.File;
import java.nio.file.Files;
public class ReadJsonFile {
public static void main(String[] args) {
try {
// 创建File对象
File jsonFile = new File("path/to/json/file.json");
// 读取文件内容并转换为字符串
String jsonString = new String(Files.readAllBytes(jsonFile.toPath()));
// 将字符串转换为JSON对象
JSONObject jsonObject = new JSONObject(jsonString);
// 访问JSON对象中的数据
String name = jsonObject.getString("name");
int age = jsonObject.getInt("age");
System.out.println("Name: " + name);
System.out.println("Age: " + age);
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
阅读全文
相关推荐
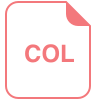
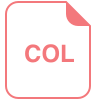
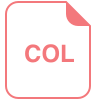
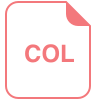
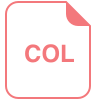
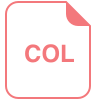
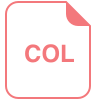
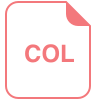
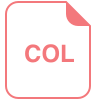
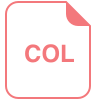
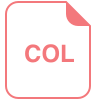
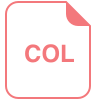
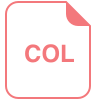
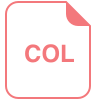

