哈希表怎么做,请用c语言进行演示
时间: 2024-10-27 20:10:06 浏览: 14
哈希表,也叫散列表,是一种常用的数据结构,通过键值对的方式存储数据。它利用哈希函数将任意大小的输入转换成固定大小的地址,实现快速的插入、删除和查找操作。在C语言中,我们可以手动实现简单的哈希表,例如使用数组作为底层存储,并用线性探测法处理冲突(当多个键映射到同一个位置的情况)。
以下是一个基本的哈希表实现示例:
```c
#include <stdio.h>
#include <stdlib.h>
#define TABLE_SIZE 10 // 哈希表大小
typedef struct Entry {
int key; // 键
int value; // 值
} Entry;
// 哈希函数,这里简单地取模运算
int hash_func(int key) {
return key % TABLE_SIZE;
}
// 哈希表节点
typedef struct HashTable {
Entry entries[TABLE_SIZE]; // 存储区
int count; // 当前元素个数
} HashTable;
HashTable *create_table() {
HashTable *table = (HashTable*)malloc(sizeof(HashTable));
table->count = 0;
return table;
}
void insert(HashTable *table, int key, int value) {
int index = hash_func(key);
while (table->entries[index].key != 0 && table->entries[index].key != key) {
if (table->entries[index].key > key)
index = (index + 1) % TABLE_SIZE;
else
index = (index - 1) % TABLE_SIZE;
}
table->entries[index].key = key;
table->entries[index].value = value;
table->count++;
}
int search(HashTable *table, int key) {
int index = hash_func(key);
while (table->entries[index].key != 0 && table->entries[index].key != key)
index = (index + 1) % TABLE_SIZE;
return table->entries[index].key == key ? table->entries[index].value : -1;
}
void destroy_table(HashTable *table) {
free(table);
}
int main() {
HashTable *table = create_table();
insert(table, 5, "apple");
insert(table, 8, "banana");
insert(table, 10, "cherry");
printf("Search for 5: %d\n", search(table, 5)); // 输出 "apple"
printf("Search for 12: %d\n", search(table, 12)); // 输出 "-1" (未找到)
destroy_table(table);
return 0;
}
```
注意,这是一个非常基础的哈希表实现,实际应用中可能需要考虑更多细节,如负载因子、开放寻址法等,以及更复杂的冲突解决策略。此外,上述代码并没有处理键冲突时的动态扩容和缩容。
阅读全文
相关推荐

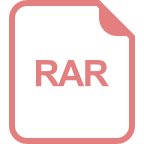
















