React实现最完整的百度搜索框
时间: 2023-08-12 13:52:06 浏览: 45
要实现一个最完整的百度搜索框,需要考虑以下几个方面:
1. 输入框的交互:包括用户输入时的提示和自动完成功能。
2. 搜索按钮的交互:包括点击搜索按钮后的搜索操作和搜索结果的展示。
3. 样式设计:包括输入框和按钮的样式,以及搜索结果的排版和样式。
在React中实现百度搜索框,可以使用antd组件库中的Input和Button组件来实现输入框和按钮的样式,同时可以使用antd的AutoComplete组件来实现自动完成功能。具体实现步骤如下:
1. 安装antd组件库:
```
npm install antd --save
```
2. 引入antd组件:
```
import { Input, Button, AutoComplete } from 'antd';
```
3. 在组件中使用antd组件:
```
const { Option } = AutoComplete;
function SearchBox() {
const [options, setOptions] = useState([]);
const handleSearch = (value) => {
setOptions(
!value ? [] : [
{ value },
{ value: value + '1' },
{ value: value + '2' },
],
);
};
const onSelect = (value) => {
console.log('onSelect', value);
};
return (
<AutoComplete
dropdownMatchSelectWidth={252}
style={{ width: 300 }}
options={options}
onSelect={onSelect}
onSearch={handleSearch}
>
<Input.Search size="large" placeholder="input here" enterButton />
</AutoComplete>
);
}
```
4. 添加搜索按钮的点击事件,实现搜索功能:
```
const [searchText, setSearchText] = useState('');
const handleSearch = () => {
window.location.href = `https://www.baidu.com/s?wd=${searchText}`;
};
<Input.Search
size="large"
placeholder="input here"
enterButton="Search"
value={searchText}
onChange={(e) => setSearchText(e.target.value)}
onSearch={handleSearch}
/>
```
5. 样式设计:可以使用antd提供的样式或自定义样式来实现自己想要的样式。
完整代码如下:
```
import { Input, Button, AutoComplete } from 'antd';
import 'antd/dist/antd.css';
import './App.css';
import React, { useState } from 'react';
const { Option } = AutoComplete;
function SearchBox() {
const [options, setOptions] = useState([]);
const [searchText, setSearchText] = useState('');
const handleSearch = () => {
window.location.href = `https://www.baidu.com/s?wd=${searchText}`;
};
const onSelect = (value) => {
setSearchText(value);
};
return (
<AutoComplete
dropdownMatchSelectWidth={252}
style={{ width: 300 }}
options={options}
onSelect={onSelect}
onSearch={() => {}}
>
<Input.Search
size="large"
placeholder="input here"
enterButton="Search"
value={searchText}
onChange={(e) => setSearchText(e.target.value)}
onSearch={handleSearch}
/>
</AutoComplete>
);
}
function App() {
return (
<div className="App">
<SearchBox />
</div>
);
}
export default App;
```
相关推荐
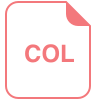
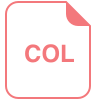
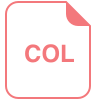
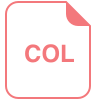
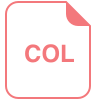









