c语言根据输入值通过银行家算法输出全部安全序列
时间: 2023-12-13 17:05:42 浏览: 106
银行家算法是一种用于避免死锁的算法,它可以判断系统是否处于安全状态。如果系统处于安全状态,那么可以为进程分配资源,否则就需要等待。以下是根据输入值通过银行家算法输出全部安全序列的 C 语言代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_PROCESS 10
#define MAX_RESOURCE 10
int main() {
int available[MAX_RESOURCE];
int allocation[MAX_PROCESS][MAX_RESOURCE];
int need[MAX_PROCESS][MAX_RESOURCE];
int max[MAX_PROCESS][MAX_RESOURCE];
int finish[MAX_PROCESS];
int work[MAX_RESOURCE];
int sequence[MAX_PROCESS];
int num_processes, num_resources;
int i, j, k, count = 0, safe = 0;
printf("Enter the number of processes: ");
scanf("%d", &num_processes);
printf("Enter the number of resources: ");
scanf("%d", &num_resources);
// input available resources
printf("Enter the available resources:\n");
for (i = 0; i < num_resources; i++) {
scanf("%d", &available[i]);
}
// input allocation matrix
printf("Enter the allocation matrix:\n");
for (i = 0; i < num_processes; i++) {
for (j = 0; j < num_resources; j++) {
scanf("%d", &allocation[i][j]);
}
}
// input max matrix
printf("Enter the max matrix:\n");
for (i = 0; i < num_processes; i++) {
for (j = 0; j < num_resources; j++) {
scanf("%d", &max[i][j]);
}
}
// calculate need matrix
for (i = 0; i < num_processes; i++) {
for (j = 0; j < num_resources; j++) {
need[i][j] = max[i][j] - allocation[i][j];
}
}
// initialize finish array to 0
for (i = 0; i < num_processes; i++) {
finish[i] = 0;
}
// initialize work array
for (i = 0; i < num_resources; i++) {
work[i] = available[i];
}
// find a safe sequence
while (count < num_processes) {
safe = 0;
for (i = 0; i < num_processes; i++) {
if (!finish[i]) {
for (j = 0; j < num_resources; j++) {
if (need[i][j] > work[j]) {
break;
}
}
if (j == num_resources) {
for (k = 0; k < num_resources; k++) {
work[k] += allocation[i][k];
}
sequence[count] = i;
finish[i] = 1;
count++;
safe = 1;
}
}
}
if (!safe) {
printf("System is not in safe state.\n");
return 0;
}
}
// print safe sequence
printf("Safe sequence: ");
for (i = 0; i < num_processes; i++) {
printf("%d ", sequence[i]);
}
printf("\n");
return 0;
}
```
在上述代码中,输入部分包括可用资源矩阵、分配矩阵和最大需求矩阵,然后计算需要矩阵。然后,使用银行家算法来找到一个安全序列。如果找到了一个安全序列,则输出该序列;否则,输出“系统不处于安全状态”。
阅读全文
相关推荐
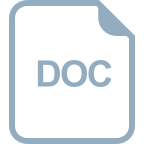
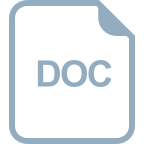
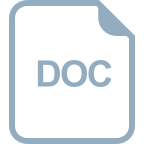

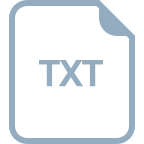
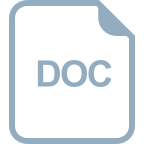
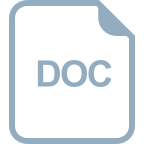
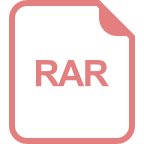
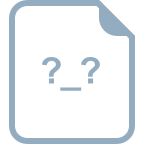
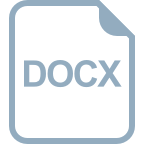
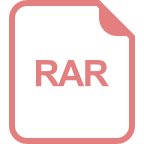
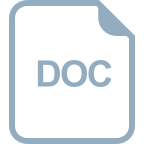
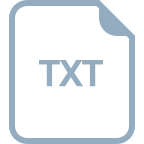
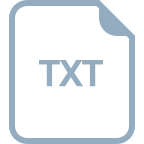
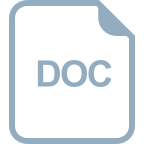
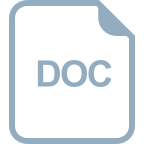


