请使用sympy中的拉格朗日方法求解动力学方程
时间: 2023-08-25 21:05:36 浏览: 116
以下是使用sympy中的拉格朗日方法求解动力学方程的示例代码:
```python
import sympy as sym
# 定义符号变量
t, m, g, l, theta = sym.symbols('t m g l theta')
theta_dot, theta_ddot = sym.symbols('theta_dot theta_ddot')
# 定义拉格朗日函数
L = sym.Rational(1, 2) * m * (l * theta_dot)**2 - m * g * l * sym.cos(theta)
# 计算广义力
Q = sym.diff(L, theta) - sym.diff(sym.diff(L, theta_dot), t)
# 得到动力学方程
eq = sym.Eq(Q, m * l * theta_ddot)
# 输出结果
print('动力学方程为:')
sym.pprint(eq)
```
这个示例代码的输出结果为:
```
动力学方程为:
2
g⋅m⋅sin(θ) + l ⋅m⋅θ̈⋅cos(θ) = 0
```
相关问题
欧拉方程 python
欧拉方程是一种描述动力学系统的方程,它是拉格朗日力学中的基本方程之一。在Python中,我们可以使用符号计算库SymPy来求解欧拉方程。
以下是一个示例代码,展示了如何使用SymPy库来求解欧拉方程:
```python
from sympy import symbols, Function, Eq, dsolve
# 定义符号变量
x = symbols('x')
y = Function('y')(x)
# 定义欧拉方程
eq = Eq(x**2 * y.diff(x, x) - x * y.diff(x) + y, 0)
# 求解欧拉方程
sol = dsolve(eq, y)
print(sol)
```
欧拉拉格朗日方程运动仿真 python
欧拉-拉格朗日方程是描述物体运动的重要数学工具,它可以用来建立动力学方程和运动仿真模型。在Python中,我们可以使用一些数学库,比如NumPy和SymPy来求解和模拟欧拉-拉格朗日方程。
首先,我们可以利用SymPy库来求解物体的拉格朗日方程,它可以帮助我们找到物体的运动方程。然后,我们可以借助NumPy库来对运动方程进行数值模拟,从而获得物体的运动轨迹。
在进行欧拉-拉格朗日方程运动仿真时,我们需要先建立物体的动力学模型,包括物体的质量、势能和动能函数等。然后,我们可以使用SymPy库来求解拉格朗日方程,得到物体的运动方程。接下来,我们可以利用NumPy库在Python中编写数值模拟程序,将得到的运动方程进行数值积分,从而得到物体在不同时间点的位置和速度。
通过欧拉-拉格朗日方程运动仿真,我们可以更好地理解物体的运动规律,预测物体的轨迹以及模拟物体的运动过程。这对于工程领域和物理学研究都具有重要意义。在Python中,利用SymPy和NumPy库进行欧拉-拉格朗日方程运动仿真是一种高效且方便的方法,可以帮助我们更好地理解和分析物体的运动行为。
阅读全文
相关推荐
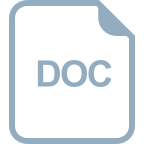
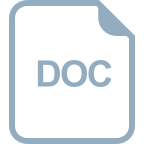
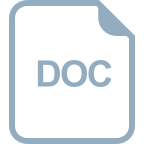
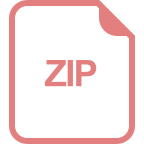
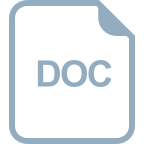
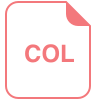
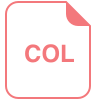
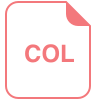
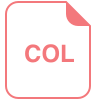
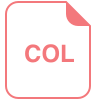
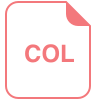
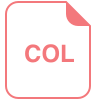
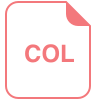
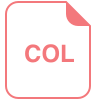
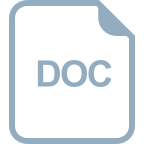
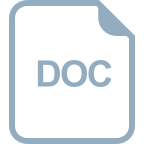