python 根据文件路径一级一级创建文件夹
时间: 2023-11-08 11:09:25 浏览: 122
可以使用Python内置的os模块中的mkdir()函数来创建文件夹。如果需要一级一级创建文件夹,可以使用os.path.join()函数构造路径,然后逐级创建文件夹。
以下是一个示例代码:
```python
import os
# 文件路径
file_path = "/home/user/documents/folder1/folder2/folder3"
# 使用os.path.join()函数构造路径
path_list = file_path.split(os.sep)
current_path = ""
for path in path_list:
current_path = os.path.join(current_path, path)
# 如果文件夹不存在则创建
if not os.path.exists(current_path):
os.mkdir(current_path)
```
在上面的代码中,我们首先使用split()函数将文件路径分割成单独的文件夹名称,然后使用os.path.join()函数逐级构建路径。在每个级别上,我们检查文件夹是否存在,如果不存在则使用mkdir()函数创建文件夹。
相关问题
写一段python脚本,提取指定路径下所有文件和子文件夹中所有文件列表,首先提取所有文件夹路径,若路径中含有static字符,则仅提取static文件夹下的一级子文件夹,并将该路径名放在excel中,
当然可以。这里是一个简单的Python脚本,使用os模块和pandas库来完成这个任务:
```python
import os
import pandas as pd
def extract_files(path, excel_writer):
# 初始化数据列表
file_data = []
# 遍历指定路径下的所有文件和子目录
for root, dirs, files in os.walk(path):
if 'static' in dirs: # 检查是否包含'static'子目录
sub_dir_path = os.path.join(root, 'static') # 获取静态目录路径
for dir_name in dirs:
if dir_name == 'static':
sub_dirs = [os.path.join(sub_dir_path, d) for d in os.listdir(sub_dir_path)] # 提取一级子目录路径
for sub_dir in sub_dirs:
file_data.append([sub_dir]) # 将路径添加到数据列表中
# 创建DataFrame并写入Excel
df = pd.DataFrame(file_data, columns=['File Paths'])
df.to_excel(excel_writer, index=False)
# 使用pandas的ExcelWriter创建一个Excel writer对象
with pd.ExcelWriter('file_paths.xlsx', engine='xlsxwriter') as writer:
extract_files('/path/to/start/directory', writer) # 替换为您需要开始提取的目录路径
```
把这个脚本保存为`extract_files.py`,然后运行它,它会在指定的起点目录下查找名为`static`的子目录,并提取出其中的所有一级子目录路径,将其写入名为`file_paths.xlsx`的Excel文件。
如何打开python文件上一级的文件夹下的名为'a'的文件
在Python中,如果你已经打开了一个文件,想要访问上一级文件夹并打开名为`a`的文件,你可以使用`os`模块中的`os.path.abspath()`函数获取当前文件的绝对路径,然后利用`os.path.dirname()`来获取上一级目录,接着再组合这个新路径和`a`的文件名。这里是一个例子:
```python
import os
# 获取当前文件的绝对路径
current_path = os.path.abspath(__file__)
# 计算上一级目录
parent_dir = os.path.dirname(current_path)
# 组合新的完整路径(假设'a'文件在上一级同名)
target_file = os.path.join(parent_dir, 'a')
try:
with open(target_file, 'r') as file_a:
# 打开并操作'a'文件
content = file_a.read()
print(content)
except FileNotFoundError:
print(f"文件'{target_file}'未找到.")
```
这段代码首先获取了当前脚本的路径,然后创建了一个指向上一级目录的路径。然后尝试打开并读取名为`a`的文件。如果`a`文件不存在,则会捕获`FileNotFoundError`。
阅读全文
相关推荐
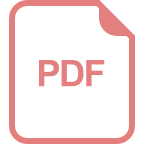
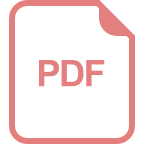
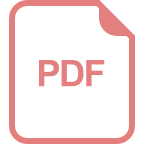
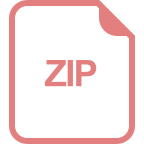
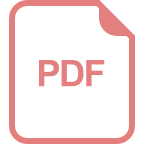
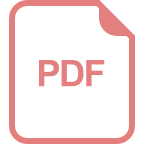
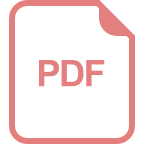
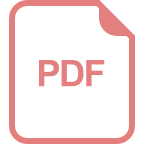
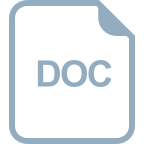
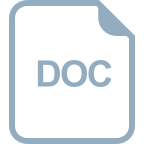
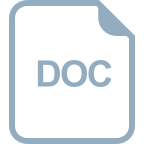
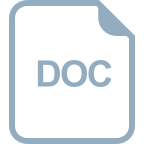
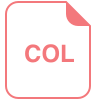
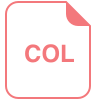
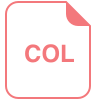