用c语言编写一个平行四边形
时间: 2024-12-08 19:21:50 浏览: 14
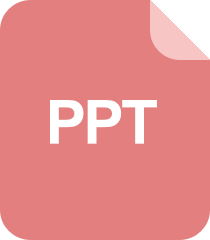
用流程图表示算法-c语言程序设计
在C语言中,要编写一个表示平行四边形的结构体,你可以首先创建一个名为`Rectangle`或`Parallelogram`的结构体,包含长和宽等属性。这里是一个简单的例子:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义平行四边形结构体
typedef struct {
int length; // 长度
int width; // 宽度
} Parallelogram;
// 初始化平行四边形的函数
Parallelogram* create_parallelogram(int len, int wid) {
Parallelogram* p = (Parallelogram*)malloc(sizeof(Parallelogram));
if (p != NULL) {
p->length = len;
p->width = wid;
} else {
printf("Memory allocation failed.\n");
return NULL;
}
return p;
}
// 显示平行四边形信息的函数
void display(Parallelogram* p) {
printf("Length: %d\n", p->length);
printf("Width: %d\n", p->width);
}
int main() {
Parallelogram* parallelogram = create_parallelogram(5, 7); // 创建一个平行四边形
if (parallelogram) {
display(parallelogram); // 展示平行四边形信息
free(parallelogram); // 释放内存
}
return 0;
}
```
在这个例子中,我们定义了一个表示平行四边形的结构体,并提供了一些基本操作,如初始化和显示。然而,由于C语言本身并不支持直接的多态,所以这个结构体的行为不会因实例的不同而改变。
阅读全文
相关推荐
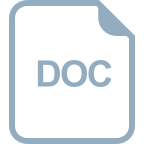
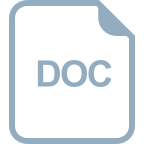





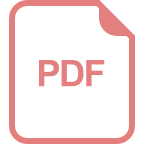
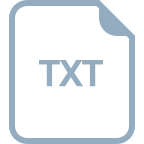


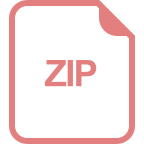
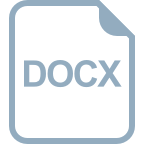
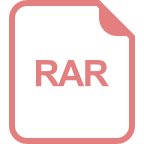
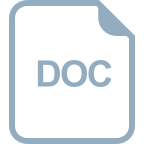
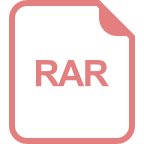
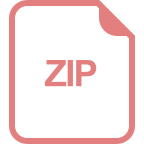
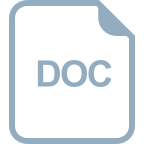