C语言借助快速排序的思想,在一组无序的记录中查找给定关键字的值等于key的记录,设此记录存放于数组 r [ 1... n ]中。若查找成功,则返回在 r数组中的位置,否则显示"not find"信息。
时间: 2024-02-28 19:55:11 浏览: 131
好的,我可以为您编写一个基于快速排序思想的查找算法。以下是C语言代码实现:
```
#include <stdio.h>
int partition(int r[], int low, int high)
{
int pivot = r[low]; // 以第一个元素作为基准值
while (low < high)
{
while (low < high && r[high] >= pivot)
high--;
r[low] = r[high];
while (low < high && r[low] <= pivot)
low++;
r[high] = r[low];
}
r[low] = pivot;
return low;
}
int quickSearch(int r[], int low, int high, int key)
{
if (low <= high)
{
int pivotIndex = partition(r, low, high);
if (r[pivotIndex] == key) // 找到了关键字
return pivotIndex;
else if (r[pivotIndex] > key) // 在左半部分继续查找
return quickSearch(r, low, pivotIndex - 1, key);
else // 在右半部分继续查找
return quickSearch(r, pivotIndex + 1, high, key);
}
else // 没有找到
return -1;
}
int main()
{
int r[] = {10, 7, 8, 9, 1, 5};
int n = sizeof(r) / sizeof(r[0]);
int key = 8;
int index = quickSearch(r, 0, n - 1, key);
if (index == -1)
printf("not find\n");
else
printf("The element is at index %d\n", index);
return 0;
}
```
在这个算法中,我们使用了快速排序的思想,将数组分成左右两个部分,然后递归地在其中一个部分中查找关键字,直到找到或者没有找到为止。如果最终没有找到,就返回 -1,否则返回关键字在数组中的位置。
希望这个算法对您有帮助!
阅读全文
相关推荐
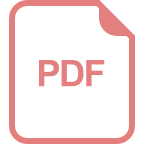
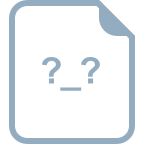


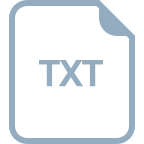
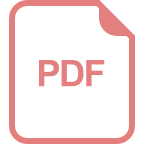
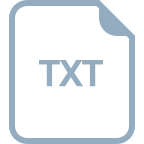
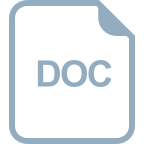
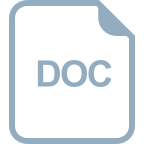
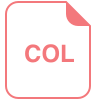
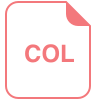
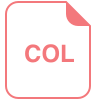




