帮我用鸢尾花(Iris)数据集中的两个特征在python环境下用五折交叉方法实现线性可分SVM,并可视化数据点和分类边界,再计算准确率
时间: 2024-10-06 19:05:43 浏览: 17
在Python环境下,我们可以使用Scikit-learn库中的`Iris`数据集和`SVC`(支持向量机)来进行线性可分的分类。这里我们将使用两个特征(例如花瓣长度(petal length)和花瓣宽度(petal width))进行五折交叉验证,并可视化结果。以下是步骤:
首先,安装必要的库:
```bash
pip install numpy pandas scikit-learn matplotlib seaborn
```
然后,导入所需的模块并加载数据:
```python
import numpy as np
import pandas as pd
from sklearn import datasets
from sklearn.model_selection import train_test_split, cross_val_score, KFold
from sklearn.svm import SVC
from sklearn.metrics import accuracy_score, confusion_matrix
import matplotlib.pyplot as plt
import seaborn as sns
# 加载Iris数据集
iris = datasets.load_iris()
X = iris.data[:, [0, 2]] # 选择前两个特征
y = iris.target
```
接下来,分割数据并创建训练集和测试集:
```python
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
```
选择五折交叉验证并使用线性SVM模型:
```python
cv = KFold(n_splits=5, shuffle=True, random_state=42)
svm = SVC(kernel='linear') # 使用线性核函数
scores = cross_val_score(svm, X_train, y_train, cv=cv)
mean_accuracy = scores.mean()
print("五折交叉验证平均准确率:", mean_accuracy)
svm.fit(X_train, y_train)
```
评估模型并在测试集上计算准确率:
```python
y_pred = svm.predict(X_test)
accuracy = accuracy_score(y_test, y_pred)
print("测试集准确率:", accuracy)
```
最后,绘制数据点和分类边界:
```python
def plot_data_and_boundary(X, y, model, title):
plt.figure(figsize=(8, 6))
plt.scatter(X[y == 0, 0], X[y == 0, 1], label='Setosa', color='blue')
plt.scatter(X[y == 1, 0], X[y == 1, 1], label='Versicolor', color='green')
plt.scatter(X[y == 2, 0], X[y == 2, 1], label='Virginica', color='red')
x_min, x_max = X[:, 0].min() - 1, X[:, 0].max() + 1
y_min, y_max = X[:, 1].min() - 1, X[:, 1].max() + 1
xx, yy = np.meshgrid(np.linspace(x_min, x_max, 100),
np.linspace(y_min, y_max, 100))
Z = model.predict(np.c_[xx.ravel(), yy.ravel()])
Z = Z.reshape(xx.shape)
plt.contourf(xx, yy, Z, alpha=0.3, cmap=plt.cm.PuBu_r)
plt.xlabel('Petal Length')
plt.ylabel('Petal Width')
plt.title(title)
plt.legend(loc='upper left')
plt.show()
plot_data_and_boundary(X_test, y_test, svm, "Linear SVM Decision Boundaries")
```
至此,你就完成了鸢尾花数据集的二维特征下线性可分SVM分类,以及数据可视化和准确率计算。
相关推荐
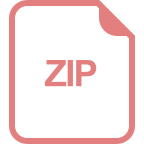
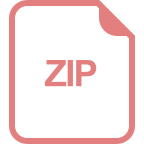
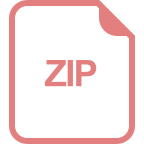














