用冒泡法对10个随机产生的数升序排序。(函数实现)
时间: 2024-05-05 19:21:38 浏览: 65
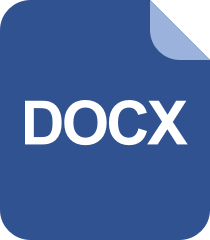
随机产生10个数 冒泡排序.docx
可以使用以下的冒泡排序算法进行实现:
```c++
void bubbleSort(int arr[], int n) {
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
int temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
}
```
其中`arr`是要排序的数组,`n`是数组的长度。该算法会在`n`次迭代中完成排序,每次迭代会比较相邻的两个元素,如果需要交换,就进行交换。最终,数组会按照升序排列。
你可以在主函数中调用该函数,并生成10个随机数作为参数传入。例如:
```c++
#include <iostream>
#include <cstdlib>
#include <ctime>
using namespace std;
void bubbleSort(int arr[], int n);
int main() {
srand(time(NULL));
int arr[10];
for (int i = 0; i < 10; i++) {
arr[i] = rand() % 100;
}
bubbleSort(arr, 10);
for (int i = 0; i < 10; i++) {
cout << arr[i] << " ";
}
cout << endl;
return 0;
}
```
这里使用了`rand()`函数生成随机数,每次运行程序生成的数都不同。
阅读全文
相关推荐

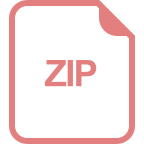
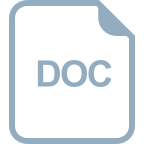


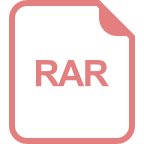
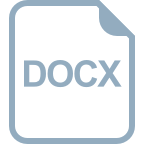
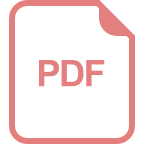
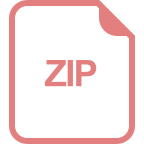
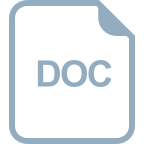
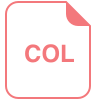
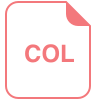
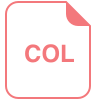
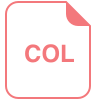
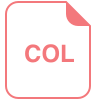
