DLT相机标定算法pytorch
时间: 2024-10-12 20:02:15 浏览: 34
DLT(Direct Linear Transformation)是一种经典的相机标定方法,它结合了特征匹配和线性代数。在PyTorch中进行相机标定,通常涉及以下几个步骤:
1. **特征检测与描述**:首先使用OpenCV或其他库(如Dlib、Detectron2等)在图像上检测特征点,并计算它们的描述符。
2. **匹配关键点**:将两幅或多幅图像中的特征点进行配对,比如通过Brute Force matcher或者更高效的Flann Matcher。
3. **构建系统矩阵**:对于每一对匹配的特征,使用DLT公式构造方程组。系统矩阵通常包括内参矩阵(焦距、主点坐标)、旋转和平移矩阵。
4. **估计相机参数**:使用PyTorch的优化函数(如`torch.optim`模块)解决这个线性系统,找到最优解,即相机参数。
5. **验证与调整**:检查标定结果的合理性,例如检查是否满足几何约束,然后如有需要,可能还需要迭代优化。
以下是一个简化的示例代码片段,展示如何在PyTorch环境中实现基本的相机标定流程:
```python
import torch
from torchvision import transforms
from skimage.feature import match_descriptors
from cv2 import findChessboardCorners
# ... (特征检测、描述子计算)
# 假设我们有两幅图像I0和I1以及对应的特征点
features0, descriptors0 = ..., ...
features1, descriptors1 = ..., ...
# 匹配特征
matches = match_descriptors(descriptors0, descriptors1)
good_matches = matches[matches.distance < threshold]
# 构建系统矩阵
if good_matches.shape[0] > min_match_count:
# 假设我们已经知道了棋盘格的结构
corners0, _ = findChessboardCorners(features0, ...)
corners1 = features1[good_matches[:, 1].astype(int)]
# 使用DLT公式
homography, _ = cv2.findHomography(corners0, corners1, method=cv2.RANSAC)
# 将homography转换为OpenCV的相机矩阵
K, R, t, E, F = cv2.decomposeHomographyMat(homography)
# 优化过程,这里只是一个简单的示例
params = torch.tensor([K[0, 0], K[1, 1], K[0, 2], K[1, 2],
R.reshape(-1), t.reshape(-1)]).float()
optimizer = torch.optim.LBFGS(params, lr=1e-2)
def closure():
optimizer.zero_grad()
error = ... # 根据匹配的质量计算误差
loss = error.sum()
loss.backward()
return loss
optimizer.step(closure)
阅读全文
相关推荐
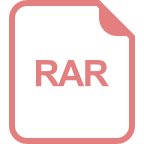
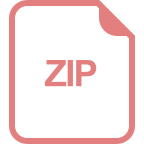
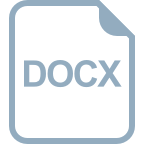

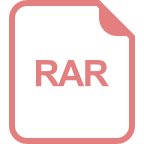
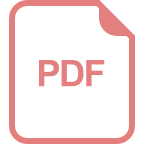
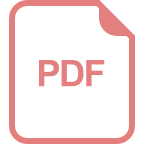
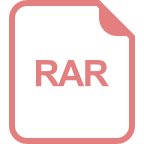
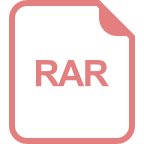
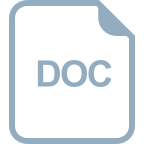
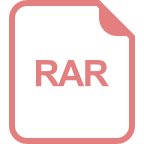
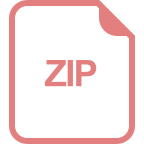
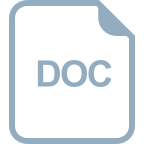
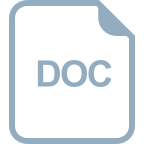




