解释一下下面这段代码import java.util.Stack; public class LR0Parser { // 定义LR(0)分析表 private final int[][] actionTable = { {2, 0, 3, 0, 0}, {0, 0, 0, 5, -1}, {2, 0, 3, 0, 0}, {-2, -2, -2, -2, -2}, {-1, -1, -1, 5, -1}, {2, 0, 3, 0, 0, 0}, {-4, -4, -4, -4, -4}, {-3, -3, -3, -3, -3} }; private final int[][] gotoTable = { {1, 4}, {0, 0}, {6, 4}, {0, 0}, {0, 0}, {0, 7}, {0, 0}, {0, 0} }; private final String[] grammar = {"E->E+T", "E->T", "T->(E)", "T->a"}; public boolean parse(String input) { Stack<Integer> stateStack = new Stack<>(); Stack<Character> symbolStack = new Stack<>(); stateStack.push(0); int index = 0; while (index < input.length()) { int state = stateStack.peek(); char symbol = input.charAt(index); int action = getAction(state, symbol); if (action > 0) {//移入 stateStack.push(action); symbolStack.push(symbol); index++; } else if (action < 0) {//规约 String production = grammar[-action - 1]; char nonTerminal = production.charAt(0); int newState = gotoTable[state][nonTerminal - 'E']; stateStack.push(newState); symbolStack.push(nonTerminal); } else { return false; } } return true; } private int getAction(int state, char symbol) { switch (symbol) { case '(': return actionTable[state][0]; case ')': return actionTable[state][1]; case 'a': return actionTable[state][2]; case '+': return actionTable[state][3]; case '$': return actionTable[state][5]; default: return -1; } } public static void main(String[] args) { LR0Parser parser = new LR0Parser(); boolean success = parser.parse("a+(a)"); if (success) { System.out.println("分析成功"); } else { System.out.println("分析失败"); } } }
时间: 2024-04-07 14:27:59 浏览: 11
这段代码实现了一个 LR(0)语法分析器,用于判断输入字符串是否符合给定的文法。其中,LR(0)分析表由 actionTable 和 gotoTable 两个二维数组表示,分别对应了移入和规约的状态转移。grammar 数组定义了文法规则,parse 方法实现了语法分析的具体过程,主函数中调用了 parse 方法对字符串 "a+(a)" 进行分析。
具体来说,程序通过维护两个栈 stateStack 和 symbolStack 来实现语法分析。stateStack 存储当前的状态,而 symbolStack 存储当前已读入的符号。在读入新的符号后,程序根据当前状态和读入符号在 actionTable 中查找相应的操作。如果是移入操作,将状态和符号入栈;如果是规约操作,将栈中符号按照对应的文法规则合并,并根据 gotoTable 中指定的状态转移进入新状态;如果操作为 0,表示分析失败,返回 false。最终,如果结束符号 $ 能够成功被规约,说明输入字符串符合给定的文法,返回 true,否则返回 false。
相关问题
补全这段代码import javax.swing.*; import java.awt.*; import java.awt.event.*; import java.util.Timer; import java.util.TimerTask; public class RestReminder extends JFrame { private JLabel timeLabel; private JButton setButton; private JButton resetButton; private JTextField restTimeField; private Timer timer; public RestReminder() { // 初始化界面和组件 // 添加事件监听器 // 实现设置休息时间和提醒时间的功能 } public static void main(String[] args) { RestReminder reminder = new RestReminder(); reminder.setVisible(true); } }
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
import java.util.Timer;
import java.util.TimerTask;
public class RestReminder extends JFrame {
private JLabel timeLabel;
private JButton setButton;
private JButton resetButton;
private JTextField restTimeField;
private Timer timer;
private int restTime; // 休息时间,单位为分钟
public RestReminder() {
// 初始化界面和组件
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setTitle("休息提醒");
setSize(300, 200);
setLocationRelativeTo(null);
timeLabel = new JLabel("00:00");
timeLabel.setFont(new Font("宋体", Font.BOLD, 40));
timeLabel.setHorizontalAlignment(SwingConstants.CENTER);
setButton = new JButton("设置");
resetButton = new JButton("重置");
restTimeField = new JTextField();
restTimeField.setColumns(10);
JPanel panel = new JPanel(new GridLayout(3, 1));
panel.add(timeLabel);
JPanel buttonPanel = new JPanel(new FlowLayout());
buttonPanel.add(setButton);
buttonPanel.add(resetButton);
panel.add(buttonPanel);
JPanel restTimePanel = new JPanel(new FlowLayout());
restTimePanel.add(new JLabel("休息时间(分钟):"));
restTimePanel.add(restTimeField);
panel.add(restTimePanel);
add(panel);
// 添加事件监听器
setButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
try {
restTime = Integer.parseInt(restTimeField.getText());
startTimer();
} catch (NumberFormatException ex) {
JOptionPane.showMessageDialog(RestReminder.this,
"请输入正确的休息时间(整数)", "错误", JOptionPane.ERROR_MESSAGE);
}
}
});
resetButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
stopTimer();
timeLabel.setText("00:00");
}
});
}
// 实现设置休息时间和提醒时间的功能
private void startTimer() {
if (timer != null) {
stopTimer();
}
int delay = 1000; // 延迟1秒钟开始执行任务
int period = 60 * 1000; // 每隔1分钟执行一次任务
timer = new Timer();
timer.scheduleAtFixedRate(new TimerTask() {
int time = restTime;
@Override
public void run() {
if (time <= 0) {
JOptionPane.showMessageDialog(RestReminder.this,
"休息时间到了,请休息一下!", "休息提醒", JOptionPane.INFORMATION_MESSAGE);
time = restTime;
}
int minute = time / 60;
int second = time % 60;
timeLabel.setText(String.format("%02d:%02d", minute, second));
time--;
}
}, delay, period);
}
private void stopTimer() {
if (timer != null) {
timer.cancel();
timer = null;
}
}
public static void main(String[] args) {
RestReminder reminder = new RestReminder();
reminder.setVisible(true);
}
}
逐句分析import java.awt.;import java.awt.event.;import java.util.Date;import javax.swing.*;public class RecordAbsence extends JFrame implements ActionListener { private JLabel label1, label2; private
这段代码是一个Java程序的开头部分,它包含了一些Java类和接口的引用,以及一个继承自JFrame类并实现ActionListener接口的RecordAbsence类的定义。
具体来说,这段代码:
- 引用了java.awt、java.awt.event和java.util包中的类和接口,这些类和接口提供了与GUI、事件和日期相关的功能。
- 引用了javax.swing包中的JFrame类,这个类是一个顶级的容器窗口,用于创建GUI应用程序的主窗口。
- 定义了一个名为RecordAbsence的类,这个类继承自JFrame类并实现了ActionListener接口,这意味着它可以处理用户界面上的动作事件。
- 定义了两个私有的JLabel对象label1和label2,这些对象将在GUI中显示一些文本。
相关推荐
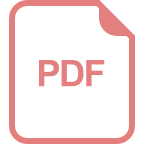













