vue3 base64转二维码
时间: 2023-07-01 22:10:55 浏览: 153
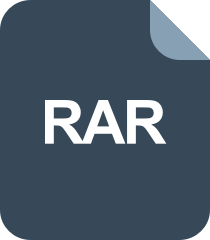
二维码转化BASE64字符串存储
你可以使用 `qrcodejs2` 库,首先安装该库:
```bash
npm install qrcodejs2
```
然后在Vue3组件中导入并使用该库:
```html
<template>
<div>
<div ref="qrcode"></div>
</div>
</template>
<script>
import QRCode from 'qrcodejs2'
export default {
mounted() {
const qrcode = new QRCode(this.$refs.qrcode, {
text: 'Hello, world!', // 这里替换为你的base64字符串
width: 256,
height: 256,
colorDark: '#000000',
colorLight: '#ffffff',
correctLevel: QRCode.CorrectLevel.H
})
}
}
</script>
```
其中 `text` 属性替换为你的base64字符串即可。
阅读全文
相关推荐
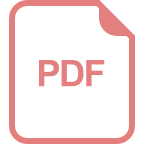






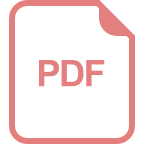
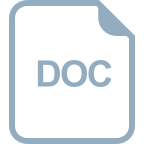







