function factorial(n){ if(n == 1 || n === 0){ return 1 } return n * factorial(n-1) } factorial(3) 详细解释这段代码的运行步骤 怎么得出6这个值
时间: 2024-04-05 07:31:41 浏览: 54
这段代码是一个使用递归方式计算阶乘的 JavaScript 函数。当函数被调用时,它接收一个参数 n,表示要计算的阶乘数。函数首先检查 n 是否等于 1 或 0,如果是,则返回 1,因为 0 和 1 的阶乘都是 1。如果 n 不等于 1 或 0,则返回 n 乘以调用自身并传入 n - 1 的结果。
当我们调用函数 `factorial(3)` 时,首先检查 3 是否等于 1 或 0,这不是,所以返回 `3 * factorial(2)`。然后,函数计算 `factorial(2)`,再次检查 2 是否等于 1 或 0,这不是,所以返回 `2 * factorial(1)`。最后,函数计算 `factorial(1)`,这个时候检查 1 是否等于 1 或 0,这是,所以返回 1。这个 1 的值被传递回 `factorial(2)`,计算结果为 `2 * 1 = 2`。这个 2 的值被传递回 `factorial(3)`,计算结果为 `3 * 2 = 6`。所以,最终的结果是 6。
相关问题
def factorial(n): if n == 0: return 1 else: return n * factorial(n-1) n = 10 print(factorial(n))
The above code defines a function named "factorial" that takes an integer "n" as input and recursively calculates and returns the factorial of "n".
The factorial of a non-negative integer "n" is defined as the product of all positive integers less than or equal to "n". For example, the factorial of 5 is 5 x 4 x 3 x 2 x 1 = 120.
The code then sets the value of "n" to 10 and calls the "factorial" function with "n" as input. The result (3628800, the factorial of 10) is printed to the console.
def factorial(n): if n == 0: return 1 else: return n * factorial(n-1)
This is a recursive function to calculate the factorial of a given number.
The factorial of a number is the product of all integers from 1 to that number. For example, the factorial of 5 is 5 x 4 x 3 x 2 x 1 = 120.
The function takes in a parameter 'n', which is the number whose factorial is to be calculated. If the number is 0, the function returns 1 (since the factorial of 0 is 1). Otherwise, it recursively calls itself with n-1 as the parameter and multiplies the result with n. This continues until n reaches 0, at which point the function returns the final result.
阅读全文
相关推荐
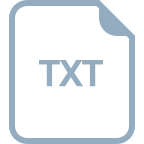
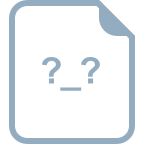
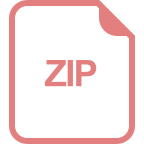










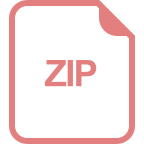
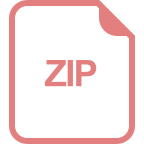
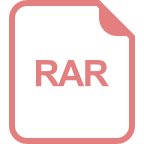
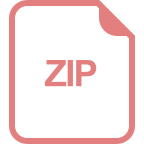