android如何实现渐变的高斯模糊
时间: 2023-09-16 17:09:59 浏览: 296
Android中可以使用RenderScript库实现高斯模糊效果,同时结合GradientDrawable实现渐变效果。具体实现步骤如下:
1.在build.gradle文件中添加RenderScript支持:
```
defaultConfig {
// ...
renderscriptTargetApi 19
renderscriptSupportModeEnabled true
}
```
2.创建RenderScript对象:
```
RenderScript rs = RenderScript.create(context);
```
3.创建高斯模糊脚本:
```
ScriptIntrinsicBlur blurScript = ScriptIntrinsicBlur.create(rs, Element.U8_4(rs));
```
4.设置高斯模糊半径:
```
blurScript.setRadius(radius);
```
其中radius是半径值,越大模糊效果越强。
5.将Bitmap转换为RenderScript的Allocation对象:
```
Allocation input = Allocation.createFromBitmap(rs, bitmap);
```
6.创建一个输出Allocation对象:
```
Allocation output = Allocation.createTyped(rs, input.getType());
```
7.在RenderScript中处理图像:
```
blurScript.setInput(input);
blurScript.forEach(output);
```
8.将处理后的图像从Allocation对象中复制到Bitmap中:
```
output.copyTo(bitmap);
```
9.使用GradientDrawable创建渐变背景:
```
GradientDrawable drawable = new GradientDrawable(GradientDrawable.Orientation.TOP_BOTTOM,
new int[] {startColor, endColor});
```
其中startColor和endColor是起始和结束颜色。
10.将Bitmap设置为View的背景:
```
view.setBackground(new BitmapDrawable(context.getResources(), bitmap));
```
完整的代码示例:
```
RenderScript rs = RenderScript.create(context);
ScriptIntrinsicBlur blurScript = ScriptIntrinsicBlur.create(rs, Element.U8_4(rs));
blurScript.setRadius(radius);
Allocation input = Allocation.createFromBitmap(rs, bitmap);
Allocation output = Allocation.createTyped(rs, input.getType());
blurScript.setInput(input);
blurScript.forEach(output);
output.copyTo(bitmap);
GradientDrawable drawable = new GradientDrawable(GradientDrawable.Orientation.TOP_BOTTOM,
new int[] {startColor, endColor});
view.setBackground(new BitmapDrawable(context.getResources(), bitmap));
```
注意:以上代码仅为示例,具体实现可能需要根据项目需求进行修改。
阅读全文
相关推荐

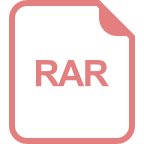
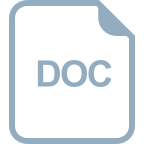
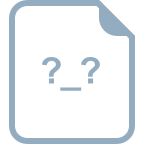
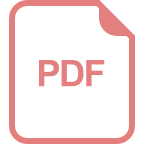
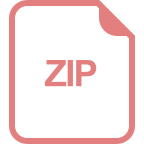
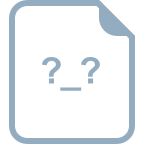
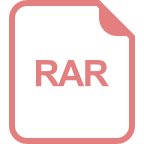
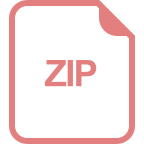
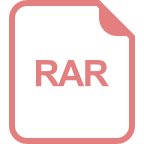
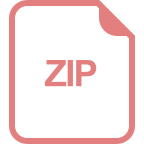
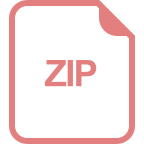
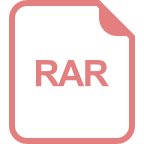
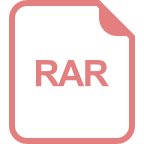
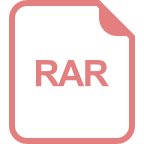