C语言实现链队列
时间: 2023-08-20 19:14:26 浏览: 57
下面是使用 C 语言实现链队列的基本代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 链队列结构体定义
typedef struct QueueNode {
int data;
struct QueueNode* next;
} QueueNode;
typedef struct {
QueueNode* front; // 队头指针
QueueNode* rear; // 队尾指针
} LinkQueue;
// 初始化链队列
void InitQueue(LinkQueue* Q) {
Q->front = Q->rear = (QueueNode*)malloc(sizeof(QueueNode));
Q->front->next = NULL;
}
// 入队操作
void EnQueue(LinkQueue* Q, int x) {
QueueNode* s = (QueueNode*)malloc(sizeof(QueueNode));
s->data = x;
s->next = NULL;
Q->rear->next = s;
Q->rear = s;
}
// 出队操作
int DeQueue(LinkQueue* Q) {
if (Q->front == Q->rear) {
printf("队列为空!\n");
exit(-1);
}
QueueNode* p = Q->front->next;
int x = p->data;
Q->front->next = p->next;
if (Q->rear == p) Q->rear = Q->front;
free(p);
return x;
}
// 判断队列是否为空
int IsEmpty(LinkQueue* Q) {
if (Q->front == Q->rear) return 1;
else return 0;
}
// 获取队列元素个数
int GetLength(LinkQueue* Q) {
int count = 0;
QueueNode* p = Q->front->next;
while (p) {
count++;
p = p->next;
}
return count;
}
// 清空队列
void ClearQueue(LinkQueue* Q) {
QueueNode* p = Q->front->next;
while (p) {
QueueNode* temp = p;
p = p->next;
free(temp);
}
Q->front->next = NULL;
Q->rear = Q->front;
}
// 输出队列元素
void PrintQueue(LinkQueue* Q) {
QueueNode* p = Q->front->next;
while (p) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
int main() {
LinkQueue Q;
InitQueue(&Q);
EnQueue(&Q, 1);
EnQueue(&Q, 2);
EnQueue(&Q, 3);
EnQueue(&Q, 4);
PrintQueue(&Q);
printf("队列长度为:%d\n", GetLength(&Q));
printf("出队元素为:%d\n", DeQueue(&Q));
printf("出队元素为:%d\n", DeQueue(&Q));
PrintQueue(&Q);
printf("队列长度为:%d\n", GetLength(&Q));
ClearQueue(&Q);
printf("清空队列后,队列长度为:%d\n", GetLength(&Q));
return 0;
}
```
在这个代码中,使用了链表来存储队列元素。其中,`front` 指针指向链队列的头结点,`rear` 指针指向链队列的尾结点。新元素入队时,将其插入链表尾部,并修改 `rear` 指针的指向。元素出队时,从链表头部取出元素,并修改 `front` 指针的指向。
相关推荐
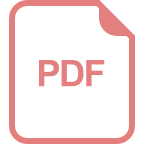












