springboot项目使用activemq
时间: 2023-08-06 20:01:44 浏览: 50
可以使用以下步骤在Spring Boot项目中集成ActiveMQ:
1. 在pom.xml中添加ActiveMQ依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-activemq</artifactId>
</dependency>
```
2. 在application.properties文件中添加ActiveMQ配置:
```properties
spring.activemq.broker-url=tcp://localhost:61616
spring.activemq.user=admin
spring.activemq.password=admin
```
3. 创建一个消息生产者:
```java
import javax.jms.ConnectionFactory;
import javax.jms.Queue;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.jms.core.JmsMessagingTemplate;
import org.springframework.stereotype.Component;
@Component
public class MessageProducer {
@Autowired
private JmsMessagingTemplate jmsMessagingTemplate;
@Autowired
private Queue queue;
public void send(String message) {
this.jmsMessagingTemplate.convertAndSend(queue, message);
}
}
```
4. 创建一个消息消费者:
```java
import javax.jms.Queue;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.jms.annotation.JmsListener;
import org.springframework.stereotype.Component;
@Component
public class MessageConsumer {
@Autowired
private Queue queue;
@JmsListener(destination = "${spring.activemq.queue-name}")
public void receive(String message) {
System.out.println("Received message: " + message);
}
}
```
5. 在应用程序中使用消息生产者发送消息:
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.CommandLineRunner;
import org.springframework.stereotype.Component;
@Component
public class MyApp implements CommandLineRunner {
@Autowired
private MessageProducer messageProducer;
@Override
public void run(String... args) throws Exception {
messageProducer.send("Hello, World!");
}
}
```
这样就可以在你的Spring Boot应用程序中使用ActiveMQ了。
相关推荐
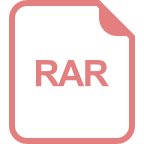
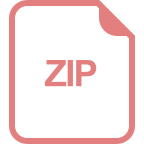














