List<Integer> aList = new ArrayList<>(Arrays.asList(1, 2, 3, 3)); List<Integer> bList = new ArrayList<>(Arrays.asList(2, 3)); 在alist中移除blist中的单个元素
时间: 2024-01-01 07:19:06 浏览: 31
好的,如果想要在`aList`中移除`bList`中的单个元素,可以使用Java8的Stream API,结合`List.removeIf()`方法来完成,代码如下:
```java
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
public class RemoveListElements {
public static void main(String[] args) {
List<Integer> aList = new ArrayList<>(Arrays.asList(1, 2, 3, 3));
List<Integer> bList = new ArrayList<>(Arrays.asList(2, 3));
// 在aList中移除bList中的单个元素
bList.forEach(aList::remove);
System.out.println(aList); // 输出:[1, 3, 3]
}
}
```
这里使用了Java8的Stream API中的`forEach()`方法,将`bList`中的每个元素作为参数传递给`aList.remove()`方法,从而实现在`aList`中移除`bList`中的单个元素。注意,这里的操作会直接修改`aList`列表,因此需要注意原列表的备份和安全性。
相关问题
List<String> temp = new ArrayList<>(Arrays.asList(s));解释一席a
这是一行Java代码,它的作用是将一个字符串s转换成一个字符串列表(List<String>),并将该列表赋值给变量temp。
具体来说,代码中使用了Arrays.asList()方法将字符串s转换成一个数组,然后将该数组作为参数传递给ArrayList的构造函数,从而创建一个包含s的字符串列表。最后,该列表被赋值给变量temp。
需要注意的是,该代码只能用于将单个字符串s转换成一个字符串列表,如果s本身已经是一个字符串列表,那么就不需要使用Arrays.asList()方法进行转换了。
method List.add(int,List<Integer>) is not applicable
This error message typically occurs when you try to call the `add` method of a `List` with an `int` as the first argument, followed by a `List<Integer>` as the second argument.
The `add` method of a `List` expects the first argument to be an `int` representing the index at which to add the element, followed by the element to be added.
To fix this error, you should change the first argument to the index at which you want to add the `List<Integer>` to the existing `List`. You can do this using the `addAll` method instead of `add`. Here's an example:
```
List<Integer> list1 = new ArrayList<>();
List<Integer> list2 = Arrays.asList(1, 2, 3);
int index = 0;
list1.addAll(index, list2);
```
This will add the elements of `list2` to `list1` at the specified index.
相关推荐
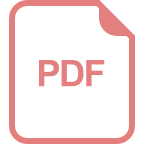
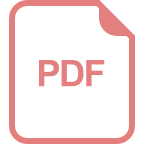












