C#实现获取远程下发的一条java命令的进程的processName并杀掉它
时间: 2024-10-28 21:10:04 浏览: 35
在C#中,你可以使用`System.Diagnostics.Process`类来获取远程计算机上运行的进程信息以及执行相关的操作。首先,你需要通过网络通信协议(如SSH、RPC等)连接到目标机器,并获取到该进程的ID(PID)。一旦得到了PID,你可以使用下面的步骤来获取进程名(ProcessName)并尝试终止它:
1. 引入必要的命名空间:
```csharp
using System;
using System.Diagnostics;
using System.Net;
using System.Net.Sockets;
using System.Management; // 如果需要查询远程Windows系统进程,需要这个命名空间
```
2. 创建一个方法来获取远程进程信息:
```csharp
public static string GetRemoteProcessName(string remoteMachine, int pid)
{
try
{
if (IsWindows(remoteMachine))
{
ManagementObjectSearcher searcher = new ManagementObjectSearcher($"SELECT Name FROM Win32_Process WHERE ProcessId={pid} AND Name LIKE '%[P]owerShell%'");
foreach (ManagementObject process in searcher.Get())
{
return process["Name"].ToString();
}
return "Not found"; // 如果找不到匹配的进程,返回默认消息
}
else // 假设是Linux环境,这里只是一个简化示例,实际可能需要使用其他库如SSH.NET
{
using (TcpClient client = new TcpClient(remoteMachine, 22)) // 使用SSH默认端口
using (StreamReader reader = new StreamReader(client.GetStream()))
{
string command = $"ps aux | grep {pid}";
byte[] responseBytes = Encoding.ASCII.GetBytes(command);
client.Send(responseBytes);
string output = reader.ReadToEnd().Trim();
// 在输出中查找进程名
string[] lines = output.Split('\n');
if (lines.Length > 0)
{
return lines[0].Split()[0];
}
else
{
return "Not found";
}
}
}
}
catch (Exception ex)
{
Console.WriteLine($"Failed to get process name: {ex.Message}");
return "Error";
}
}
private static bool IsWindows(string hostname)
{
try
{
IPHostEntry host = Dns.GetHostEntry(hostname);
return host.AddressList.Any(ip => ip.AddressFamily == AddressFamily.InterNetworkV6);
}
catch (Exception)
{
return false;
}
}
```
3. 杀死进程:
```csharp
public static void KillRemoteProcess(string remoteMachine, int pid)
{
string processName = GetRemoteProcessName(remoteMachine, pid);
if (!string.IsNullOrEmpty(processName))
{
if (IsWindows(remoteMachine))
{
// Windows 环境下直接kill进程
RemoteExecuteCommand(remoteMachine, $"taskkill /F /IM {processName} /T");
}
else
{
// Linux 环境下可能需要使用SSH或其他工具来发送shell命令
RemoteExecuteCommand(remoteMachine, $"kill -9 {pid}");
}
Console.WriteLine($"Successfully killed process named '{processName}' on {remoteMachine}");
}
else
{
Console.WriteLine($"Cannot find process with PID {pid} on {remoteMachine}");
}
}
private static void RemoteExecuteCommand(string hostname, string command)
{
// 实现异步或同步执行远程命令,这里仅提供基本框架,实际使用需填充具体的网络通信逻辑
// ...
}
```
**相关问题--:**
1. 如何处理远程连接失败的情况?
2. 对于非PowerShell的进程,如何在Windows上找到它的名字?
3. 如何安全地执行远程命令以防止恶意操作?
阅读全文
相关推荐





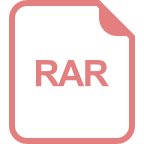


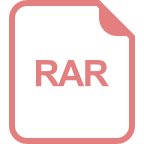
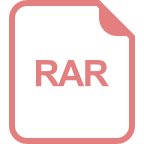



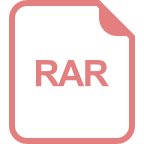

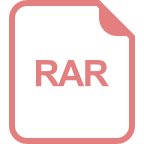

