在pyqt中如何使用QMessage
时间: 2023-07-09 16:37:16 浏览: 215
在 PyQT 中,你可以使用 QMessageBox 类来显示消息框。QMessageBox 提供了多个预定义的消息框类型,例如信息框、警告框、错误框、提问框等等。
下面是一个使用 QMessageBox 显示信息框的例子:
```python
from PyQt5.QtWidgets import QMessageBox, QApplication
import sys
app = QApplication(sys.argv)
messageBox = QMessageBox()
messageBox.setText("Hello, world!")
messageBox.setWindowTitle("Information")
messageBox.setIcon(QMessageBox.Information)
messageBox.setStandardButtons(QMessageBox.Ok)
messageBox.exec_()
```
在上面的例子中,我们首先导入了 QMessageBox 和 QApplication 类。然后,我们创建了一个 QApplication 对象并传入命令行参数。接下来,我们创建了一个 QMessageBox 对象,并设置了消息文本、标题、图标和标准按钮。最后,我们调用了 exec_() 方法来显示消息框。
你可以根据需要设置不同的消息框类型、图标、按钮等等。更多关于 QMessageBox 的使用方法和属性,可以参考 PyQt5 官方文档。
相关问题
pyqt ,QMessageBox使用
QMessageBox是一个常用的Qt对话框类,用于显示各种类型的消息和警告。以下是使用PyQt5中QMessageBox的示例代码:
```python
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow, QPushButton, QMessageBox
class Example(QMainWindow):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
self.setGeometry(300, 300, 300, 200)
self.setWindowTitle('QMessageBox Example')
button = QPushButton('Show MessageBox', self)
button.move(100, 100)
button.clicked.connect(self.showMessageBox)
def showMessageBox(self):
reply = QMessageBox.question(self, 'Message', 'Are you sure to quit?', QMessageBox.Yes | QMessageBox.No, QMessageBox.No)
if reply == QMessageBox.Yes:
QApplication.quit()
if __name__ == '__main__':
app = QApplication(sys.argv)
ex = Example()
ex.show()
sys.exit(app.exec_())
```
该示例代码通过QPushButton的clicked信号连接槽函数showMessageBox(),在该函数中调用QMessageBox.question()方法,显示一个带有Yes和No按钮的消息框。用户点击Yes按钮后,应用程序退出。
pyqt中pushbutton调用自定义函数
在PyQt中使用QPushButton调用自定义函数,您可以按照以下步骤进行操作:
1. 导入必要的模块:
```
from PyQt5.QtWidgets import QApplication, QWidget, QPushButton, QMessageBox
import sys
```
2. 创建一个QWidget窗口并添加一个QPushButton控件:
```
class MyWindow(QWidget):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
self.setGeometry(100, 100, 300, 200)
self.setWindowTitle('QPushButton Example')
self.button = QPushButton('Click me', self)
self.button.setGeometry(100, 100, 100, 30)
self.button.clicked.connect(self.show_message)
```
3. 创建一个自定义函数,该函数将在用户单击按钮时被调用:
```
def show_message(self):
QMessageBox.information(self, 'Message', 'Hello, World!')
```
4. 创建一个QApplication实例并显示窗口:
```
if __name__ == '__main__':
app = QApplication(sys.argv)
window = MyWindow()
window.show()
sys.exit(app.exec_())
```
现在,每当用户单击按钮时,将弹出一个消息框显示“Hello, World!”消息。
阅读全文
相关推荐
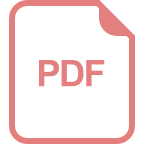
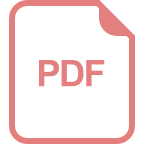
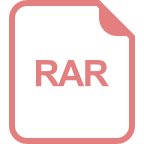
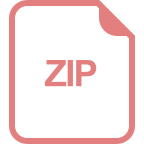
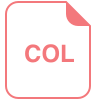
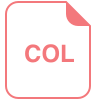










