员工信息系统数据结构c语言版,数据结构C语言_员工信息
时间: 2023-07-07 08:43:17 浏览: 44
好的,您想要实现一个员工信息系统的数据结构,以下是一个示例代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_NAME_LEN 50
#define MAX_ID_LEN 10
typedef struct Employee {
char name[MAX_NAME_LEN];
char id[MAX_ID_LEN];
int age;
float salary;
struct Employee *next;
} Employee;
Employee *head = NULL;
void addEmployee(char name[], char id[], int age, float salary) {
Employee *newEmployee = (Employee*) malloc(sizeof(Employee));
strcpy(newEmployee->name, name);
strcpy(newEmployee->id, id);
newEmployee->age = age;
newEmployee->salary = salary;
newEmployee->next = head;
head = newEmployee;
}
void printEmployee(Employee *employee) {
printf("Name: %s\n", employee->name);
printf("ID: %s\n", employee->id);
printf("Age: %d\n", employee->age);
printf("Salary: %.2f\n", employee->salary);
}
void printAllEmployees() {
Employee *current = head;
while (current != NULL) {
printEmployee(current);
current = current->next;
}
}
int main() {
addEmployee("John Smith", "123456", 30, 50000.0);
addEmployee("Mary Johnson", "789012", 25, 40000.0);
addEmployee("David Lee", "345678", 35, 60000.0);
printAllEmployees();
return 0;
}
```
在这个代码中,我们使用了链表来存储员工信息,每个员工信息包括姓名、ID、年龄、工资等属性。通过 addEmployee 函数,我们可以将新员工添加到链表的头部。通过 printAllEmployees 函数,我们可以打印出所有员工的信息。
相关推荐
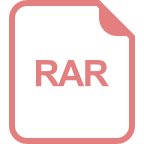














