linux c 配置文件读写实例
时间: 2023-11-11 18:04:30 浏览: 156
以下是一个简单的实例,演示如何使用 C 语言读写 Linux 下的配置文件。
假设我们有一个名为 "config.ini" 的配置文件,内容如下:
```
[database]
host = localhost
username = root
password = password123
port = 3306
[server]
ip = 127.0.0.1
port = 8080
```
我们要使用 C 语言读取这个配置文件,并将其中的值存储在内存中,以便我们在程序中使用。
首先,我们需要定义一个结构体来存储配置文件中的值:
```c
typedef struct {
char database_host[100];
char database_username[100];
char database_password[100];
int database_port;
char server_ip[100];
int server_port;
} Config;
```
接下来,我们需要编写函数来读取配置文件。以下是一个示例函数:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
void read_config_file(Config* config) {
FILE* fp;
char line[100];
char section[100];
char key[100];
char value[100];
fp = fopen("config.ini", "r");
if (fp == NULL) {
printf("Error: could not open config file.\n");
exit(1);
}
while (fgets(line, sizeof(line), fp)) {
// remove newline character
line[strcspn(line, "\n")] = 0;
if (line[0] == '[') {
// new section
strcpy(section, line);
} else {
// key-value pair
sscanf(line, "%[^=]=%s", key, value);
if (strcmp(section, "[database]") == 0) {
if (strcmp(key, "host") == 0) {
strcpy(config->database_host, value);
} else if (strcmp(key, "username") == 0) {
strcpy(config->database_username, value);
} else if (strcmp(key, "password") == 0) {
strcpy(config->database_password, value);
} else if (strcmp(key, "port") == 0) {
config->database_port = atoi(value);
}
} else if (strcmp(section, "[server]") == 0) {
if (strcmp(key, "ip") == 0) {
strcpy(config->server_ip, value);
} else if (strcmp(key, "port") == 0) {
config->server_port = atoi(value);
}
}
}
}
fclose(fp);
}
```
该函数使用 fopen() 函数打开配置文件,并使用 fgets() 函数逐行读取文件内容。对于每一行,它检查行首是否为 "[",以确定当前行是否为一个新的配置部分。如果是,它将当前部分存储在 section 变量中。否则,它使用 sscanf() 函数从当前行中提取键值对,并将其存储在相应的结构体成员中。
最后,我们可以使用以下代码将配置文件的值读入内存中:
```c
int main() {
Config config;
read_config_file(&config);
printf("database host: %s\n", config.database_host);
printf("database username: %s\n", config.database_username);
printf("database password: %s\n", config.database_password);
printf("database port: %d\n", config.database_port);
printf("server ip: %s\n", config.server_ip);
printf("server port: %d\n", config.server_port);
return 0;
}
```
该代码调用 read_config_file() 函数,并将 Config 结构体的指针传递给它。然后,它打印每个配置值的值,以确保它们已正确读入内存中。
这就是一个简单的 Linux C 配置文件读写实例。
阅读全文
相关推荐
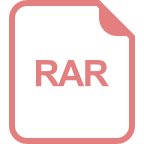







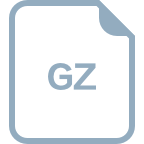






