antdv组件 + SpringBoot实现图片上传
时间: 2024-01-24 19:05:20 浏览: 25
首先,我们需要在前端使用 antdv 组件实现图片上传。可以使用 Upload 组件来实现。具体代码如下:
```
<template>
<div>
<a-upload
:action="'http://localhost:8080/api/upload'"
:headers="{Authorization: 'Bearer ' + token}"
:before-upload="beforeUpload"
:on-success="onUploadSuccess"
:show-upload-list="false"
>
<a-button icon="upload">Click to Upload</a-button>
</a-upload>
</div>
</template>
<script>
export default {
data() {
return {
token: 'your_token_here'
}
},
methods: {
beforeUpload(file) {
const isJpgOrPng = file.type === 'image/jpeg' || file.type === 'image/png';
if (!isJpgOrPng) {
this.$message.error('You can only upload JPG/PNG file!');
return false;
}
const isLt2M = file.size / 1024 / 1024 < 2;
if (!isLt2M) {
this.$message.error('Image must smaller than 2MB!');
return false;
}
return true;
},
onUploadSuccess(response) {
this.$message.success('Upload successfully!');
}
}
}
</script>
```
在这个代码中,我们通过设置 Upload 组件的属性实现了以下功能:
- 设置上传的地址为 `http://localhost:8080/api/upload`,这是后端提供的图片上传接口;
- 设置请求头中的 `Authorization` 属性为我们的 token,这是为了验证用户的身份;
- 设置上传前的验证函数 `beforeUpload`,用于检查上传的文件类型和大小是否符合要求;
- 设置上传成功后的回调函数 `onUploadSuccess`,用于在页面上提示用户上传成功。
接下来,我们需要在后端使用 SpringBoot 来接收前端上传的图片。具体代码如下:
```java
@RestController
@RequestMapping("/api")
public class UploadController {
@PostMapping("/upload")
public ResponseEntity<?> uploadFile(@RequestParam("file") MultipartFile file) {
try {
// 保存上传的文件到本地磁盘或云存储
// ...
return ResponseEntity.ok().body("Upload successfully!");
} catch (Exception e) {
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).body("Failed to upload file.");
}
}
}
```
在这个代码中,我们通过 `@PostMapping("/upload")` 注解来指定接收前端上传的图片请求。`@RequestParam("file") MultipartFile file` 表示接收一个名为 `file` 的文件参数。在接收到图片后,我们可以将其保存到本地磁盘或云存储中,并返回一个上传成功的信息给前端。如果上传过程中出现了异常,我们会返回一个 500 错误给前端。
以上就是使用 antdv 组件和 SpringBoot 来实现图片上传的基本流程。当然,具体的实现方式还会因为不同的业务需求而有所差异。
相关推荐
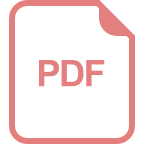
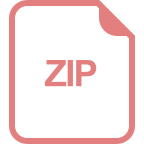
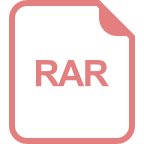














