Uni评论功能前端和后端代码
时间: 2023-08-06 19:23:14 浏览: 127
以下是一个基于 Uniapp 的简单评论功能的前端和后端代码示例:
1. 前端代码:
```
<template>
<view>
<uni-comment v-model="comment" :list="comments" @submit="submitComment"></uni-comment>
</view>
</template>
<script>
import uniComment from '@/components/uni-comment.vue'
export default {
components: {
uniComment
},
data() {
return {
comment: '',
comments: []
}
},
methods: {
submitComment() {
// 对评论内容进行过滤和验证
// 发送评论数据到后端
uni.request({
url: 'https://yourbackend.com/comments/',
method: 'POST',
data: {
content: this.comment
},
success: (res) => {
console.log(res.data)
// 更新评论列表
this.comments.push(res.data)
// 清空评论输入框
this.comment = ''
}
})
}
}
}
</script>
```
2. 后端代码(使用 Node.js 和 Express 框架):
```
const express = require('express')
const bodyParser = require('body-parser')
const app = express()
// 解析请求体中的 JSON 数据
app.use(bodyParser.json())
// 存储评论数据的数组
let comments = []
// 处理获取评论列表的请求
app.get('/comments', (req, res) => {
res.json(comments)
})
// 处理新增评论的请求
app.post('/comments', (req, res) => {
// 从请求体中获取评论内容
const content = req.body.content
// 创建新的评论对象
const newComment = {
id: comments.length + 1,
content: content,
createTime: new Date()
}
// 将新的评论对象保存到评论数组中
comments.push(newComment)
// 返回新的评论对象
res.json(newComment)
})
// 启动服务器
app.listen(3000, () => {
console.log('Server started on port 3000')
})
```
需要注意的是,上述代码只是一个简单的示例,实际中需要考虑更多的安全和数据管理问题,如对评论内容进行过滤、验证和审核,对评论数据进行存储和管理等。
阅读全文
相关推荐
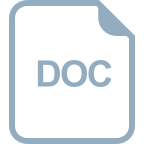
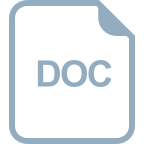
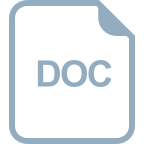
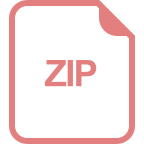
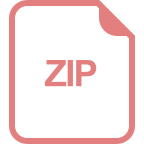
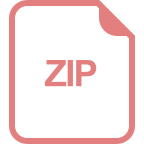
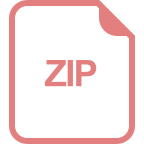
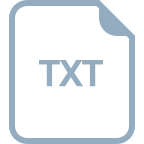
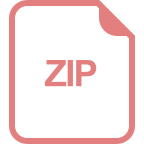
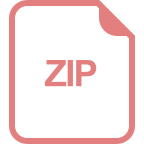
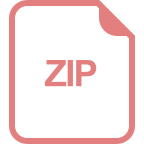
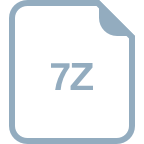
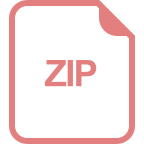
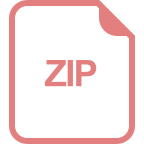
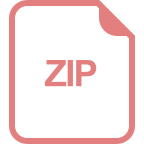
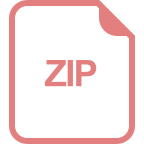

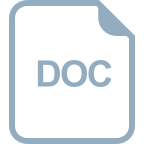