使用idea的spring框架做一个具有登录功能和增删改查的功能的通讯录源码
时间: 2023-12-10 19:41:30 浏览: 31
很抱歉,我无法为您提供完整的源代码,但我可以给您提供一些指导和建议以帮助您开始。
首先,您需要设置一个Spring Boot项目并添加所需的依赖项。您可能需要以下依赖项:
```xml
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<scope>runtime</scope>
<optional>true</optional>
</dependency>
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
</dependencies>
```
接下来,您需要创建一个简单的联系人类,如下所示:
```java
@Data
@Entity
public class Contact {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@NotBlank
private String name;
@Email
@NotBlank
private String email;
@NotBlank
private String phone;
public Contact() {}
public Contact(String name, String email, String phone) {
this.name = name;
this.email = email;
this.phone = phone;
}
}
```
然后,您需要创建一个联系人存储库接口,如下所示:
```java
@Repository
public interface ContactRepository extends JpaRepository<Contact, Long> {}
```
接下来,您需要创建一个联系人服务接口和实现,如下所示:
```java
public interface ContactService {
List<Contact> getAllContacts();
void saveContact(Contact contact);
void deleteContact(Long id);
Contact getContactById(Long id);
}
@Service
public class ContactServiceImpl implements ContactService {
@Autowired
private ContactRepository contactRepository;
@Override
public List<Contact> getAllContacts() {
return contactRepository.findAll();
}
@Override
public void saveContact(Contact contact) {
contactRepository.save(contact);
}
@Override
public void deleteContact(Long id) {
contactRepository.deleteById(id);
}
@Override
public Contact getContactById(Long id) {
Optional<Contact> optionalContact = contactRepository.findById(id);
return optionalContact.orElse(null);
}
}
```
然后,您需要创建一个控制器类,如下所示:
```java
@Controller
public class ContactController {
@Autowired
private ContactService contactService;
@GetMapping("/")
public String home(Model model) {
List<Contact> contacts = contactService.getAllContacts();
model.addAttribute("contacts", contacts);
return "home";
}
@GetMapping("/add")
public String addContactForm(Model model) {
model.addAttribute("contact", new Contact());
return "add_contact";
}
@PostMapping("/save")
public String saveContact(@ModelAttribute("contact") Contact contact) {
contactService.saveContact(contact);
return "redirect:/";
}
@GetMapping("/edit/{id}")
public String editContactForm(@PathVariable Long id, Model model) {
Contact contact = contactService.getContactById(id);
model.addAttribute("contact", contact);
return "edit_contact";
}
@PostMapping("/update/{id}")
public String updateContact(@PathVariable Long id, @ModelAttribute("contact") Contact contact) {
Contact existingContact = contactService.getContactById(id);
existingContact.setName(contact.getName());
existingContact.setEmail(contact.getEmail());
existingContact.setPhone(contact.getPhone());
contactService.saveContact(existingContact);
return "redirect:/";
}
@GetMapping("/delete/{id}")
public String deleteContact(@PathVariable Long id) {
contactService.deleteContact(id);
return "redirect:/";
}
}
```
最后,您需要创建一些前端页面,如下所示:
home.html
```html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Contacts</title>
</head>
<body>
<h1>Contacts</h1>
<table>
<tr>
<th>Name</th>
<th>Email</th>
<th>Phone</th>
<th></th>
<th></th>
</tr>
<tr th:each="contact : ${contacts}">
<td th:text="${contact.name}"></td>
<td th:text="${contact.email}"></td>
<td th:text="${contact.phone}"></td>
<td><a th:href="@{/edit/{id}(id=${contact.id})}">Edit</a></td>
<td><a th:href="@{/delete/{id}(id=${contact.id})}" onclick="return confirm('Are you sure?')">Delete</a></td>
</tr>
</table>
<p><a href="/add">Add Contact</a></p>
</body>
</html>
```
add_contact.html
```html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Add Contact</title>
</head>
<body>
<h1>Add Contact</h1>
<form th:action="@{/save}" th:object="${contact}" method="post">
<label for="name">Name:</label>
<input type="text" id="name" name="name" required th:value="${contact.name}"/><br/>
<label for="email">Email:</label>
<input type="email" id="email" name="email" required th:value="${contact.email}"/><br/>
<label for="phone">Phone:</label>
<input type="text" id="phone" name="phone" required th:value="${contact.phone}"/><br/>
<input type="submit" value="Save"/>
</form>
</body>
</html>
```
edit_contact.html
```html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Edit Contact</title>
</head>
<body>
<h1>Edit Contact</h1>
<form th:action="@{/update/{id}(id=${contact.id})}" th:object="${contact}" method="post">
<label for="name">Name:</label>
<input type="text" id="name" name="name" required th:value="${contact.name}"/><br/>
<label for="email">Email:</label>
<input type="email" id="email" name="email" required th:value="${contact.email}"/><br/>
<label for="phone">Phone:</label>
<input type="text" id="phone" name="phone" required th:value="${contact.phone}"/><br/>
<input type="submit" value="Save"/>
</form>
</body>
</html>
```
这只是一个简单的示例,但希望它能帮助您开始使用Spring框架创建通讯录应用程序。
相关推荐
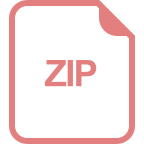
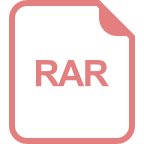














