在fastapi中如何使用进程池
时间: 2024-04-19 07:07:43 浏览: 25
在FastAPI中使用进程池可以使用Python的`concurrent.futures`模块。具体步骤如下:
1. 引入`concurrent.futures`模块。
```python
import concurrent.futures
```
2. 创建进程池。
```python
pool = concurrent.futures.ProcessPoolExecutor(max_workers=4)
```
这里创建了一个最大线程数为4的进程池。
3. 使用进程池执行任务。
```python
def some_long_running_function(arg):
# 执行需要耗时的任务
return result
@app.post("/process")
async def process(arg: str):
# 使用进程池执行任务
result = await asyncio.get_event_loop().run_in_executor(pool, some_long_running_function, arg)
return {"result": result}
```
这里定义了一个需要耗时较长时间的函数`some_long_running_function`,然后在`process`路由中使用`run_in_executor`方法将该函数交给进程池处理。这样就可以在FastAPI中使用进程池了。
相关问题
如何在python程序中间使用进程池
在Python中使用进程池可以使用multiprocessing模块。下面是一个简单的例子,演示如何在Python程序中使用进程池。
```python
import multiprocessing
def worker(num):
"""worker function"""
print('Worker:', num)
return
if __name__ == '__main__':
jobs = []
for i in range(5):
p = multiprocessing.Process(target=worker, args=(i,))
jobs.append(p)
p.start()
```
在这个例子中,我们定义了一个worker函数,它接受一个整数参数num,打印一个消息。
我们使用multiprocessing模块创建了一个进程池,然后循环创建5个进程,并将它们添加到进程池中。
最后,我们使用start方法开始每个进程的运行。
在实际应用中,我们通常会使用进程池来处理一组任务,而不是单个任务。下面是一个更完整的例子,演示如何使用进程池来并行处理多个任务:
```python
import multiprocessing
import time
def worker(num):
"""worker function"""
print('Worker:', num)
time.sleep(1)
return
if __name__ == '__main__':
# 创建进程池
pool = multiprocessing.Pool(processes=4)
# 添加任务
for i in range(10):
pool.apply_async(worker, args=(i,))
# 关闭进程池
pool.close()
# 等待所有任务完成
pool.join()
print("All tasks are done.")
```
在这个例子中,我们创建了一个拥有4个进程的进程池。然后,我们循环10次,每次向进程池添加一个任务。
最后,我们使用close方法关闭进程池,并使用join方法等待所有任务完成。在任务运行期间,我们可以看到进程池中的进程同时处理多个任务。
fastapi与多进程联用
FastAPI是一个高性能的Python Web框架,它支持异步编程和协程,因此可以与多进程联用来提高性能。在使用FastAPI时,可以使用Python内置的multiprocessing模块来创建多个进程,每个进程都可以运行FastAPI应用程序的实例。这样可以充分利用多核处理器的优势,提高应用程序的并发处理能力和响应速度。同时,由于FastAPI本身就是一个高性能的框架,与多进程联用可以进一步提高应用程序的性能表现。需要注意的是,在使用多进程时,需要注意进程之间的通信和同步,避免出现死锁等问题。
相关推荐
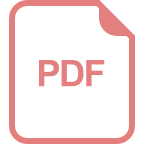
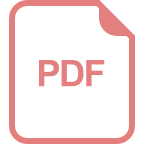
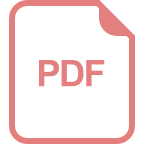
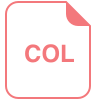
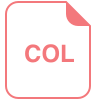
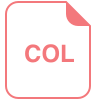
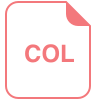
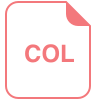







