C++GDI绘制直角坐标系,坐标原点在窗口中心,并绘制刻度
时间: 2024-04-07 12:33:25 浏览: 23
您可以使用C++的GDI(图形设备接口)来绘制直角坐标系并将坐标原点放在窗口中心。以下是一个简单的示例代码:
```cpp
#include <windows.h>
LRESULT CALLBACK WindowProc(HWND hwnd, UINT uMsg, WPARAM wParam, LPARAM lParam);
int WINAPI WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance, LPSTR lpCmdLine, int nCmdShow)
{
// 创建窗口
const wchar_t CLASS_NAME[] = L"Sample Window Class";
WNDCLASS wc = {};
wc.lpfnWndProc = WindowProc;
wc.hInstance = hInstance;
wc.lpszClassName = CLASS_NAME;
RegisterClass(&wc);
HWND hwnd = CreateWindowEx(
0,
CLASS_NAME,
L"DirectDraw Sample",
WS_OVERLAPPEDWINDOW,
CW_USEDEFAULT,
CW_USEDEFAULT,
800,
600,
nullptr,
nullptr,
hInstance,
nullptr
);
if (hwnd == nullptr)
{
return 0;
}
ShowWindow(hwnd, nCmdShow);
// 消息循环
MSG msg = {};
while (GetMessage(&msg, nullptr, 0, 0))
{
TranslateMessage(&msg);
DispatchMessage(&msg);
}
return 0;
}
LRESULT CALLBACK WindowProc(HWND hwnd, UINT uMsg, WPARAM wParam, LPARAM lParam)
{
switch (uMsg)
{
case WM_PAINT:
{
PAINTSTRUCT ps;
HDC hdc = BeginPaint(hwnd, &ps);
// 获取窗口尺寸
RECT rect;
GetClientRect(hwnd, &rect);
int width = rect.right - rect.left;
int height = rect.bottom - rect.top;
// 设置坐标原点在窗口中心
SetViewportOrgEx(hdc, width / 2, height / 2, nullptr);
// 绘制直角坐标系
MoveToEx(hdc, -width / 2, 0, nullptr);
LineTo(hdc, width / 2, 0);
MoveToEx(hdc, 0, -height / 2, nullptr);
LineTo(hdc, 0, height / 2);
// 绘制刻度
const int scaleLength = 10; // 刻度长度
const int scaleInterval = 50; // 刻度间隔
for (int x = -width / 2 + scaleInterval; x < width / 2; x += scaleInterval)
{
MoveToEx(hdc, x, -scaleLength, nullptr);
LineTo(hdc, x, scaleLength);
}
for (int y = -height / 2 + scaleInterval; y < height / 2; y += scaleInterval)
{
MoveToEx(hdc, -scaleLength, y, nullptr);
LineTo(hdc, scaleLength, y);
}
EndPaint(hwnd, &ps);
}
return 0;
case WM_DESTROY:
PostQuitMessage(0);
return 0;
default:
return DefWindowProc(hwnd, uMsg, wParam, lParam);
}
}
```
这段代码创建了一个窗口,并在窗口中心绘制了直角坐标系和刻度。您可以将该代码保存为.cpp文件,并使用Visual Studio等编译器进行编译运行。
相关推荐
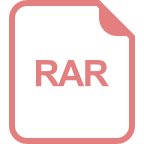














