详细讲解一下数据结构的java代码实现
时间: 2023-08-16 17:09:55 浏览: 109
当谈到数据结构的Java代码实现时,有许多不同的数据结构可供选择,例如数组、链表、栈、队列、树、图等等。我将以链表作为例子来进行讲解。
链表是一种常见的数据结构,它由一个节点的集合组成,每个节点包含两部分:数据和指向下一个节点的指针。以下是一个简单的链表的Java代码实现:
```java
// 定义链表节点类
class Node {
int data;
Node next;
// 构造函数
public Node(int data) {
this.data = data;
this.next = null;
}
}
// 定义链表类
class LinkedList {
Node head;
// 构造函数
public LinkedList() {
this.head = null;
}
// 在链表末尾添加节点
public void append(int data) {
Node newNode = new Node(data);
if (head == null) {
head = newNode;
} else {
Node current = head;
while (current.next != null) {
current = current.next;
}
current.next = newNode;
}
}
// 打印链表元素
public void printList() {
Node current = head;
while (current != null) {
System.out.print(current.data + " ");
current = current.next;
}
}
}
// 测试链表类
public class Main {
public static void main(String[] args) {
LinkedList list = new LinkedList();
// 向链表中添加节点
list.append(1);
list.append(2);
list.append(3);
// 打印链表元素
list.printList(); // 输出: 1 2 3
}
}
```
以上代码演示了如何使用Java实现一个简单的链表数据结构。你可以通过添加其他方法来实现链表的其他操作,如插入节点、删除节点等。希望这个例子能够帮助你理解数据结构的Java代码实现。
阅读全文
相关推荐
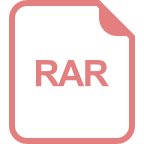
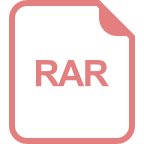
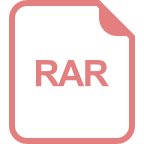
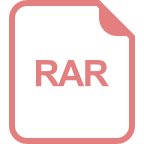
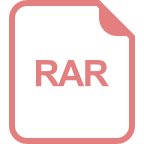
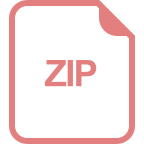
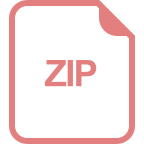
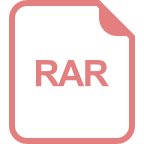
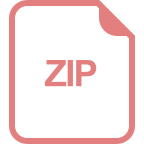
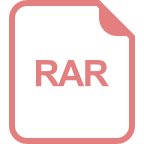
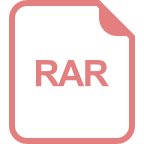
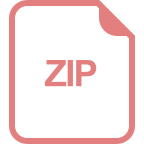
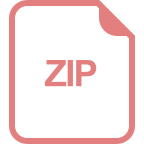
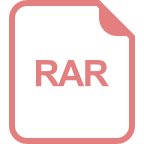