java获取windows11应用窗口标题
时间: 2023-10-19 10:27:26 浏览: 106
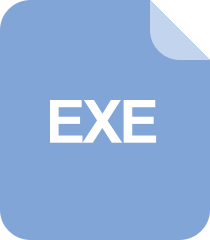
读取窗口标题
在Windows 11系统中,应用窗口的标题格式为“应用名称 - 窗口标题”,因此可以通过遍历所有系统进程,查找指定应用的进程ID,然后获取该进程的主窗口句柄,最后调用Windows API获取窗口标题的方式来实现获取Windows 11应用窗口标题的功能。
具体实现步骤如下:
1. 获取指定应用的进程ID。可以使用Java的ProcessBuilder类执行命令行获取所有正在运行的进程列表,然后遍历列表查找指定应用的进程ID,例如:
```java
public static int getProcessIdByName(String processName) throws IOException {
Process process = Runtime.getRuntime().exec("tasklist");
BufferedReader reader = new BufferedReader(new InputStreamReader(process.getInputStream()));
String line = null;
while ((line = reader.readLine()) != null) {
if (line.contains(processName)) {
String[] parts = line.split("\\s+");
return Integer.parseInt(parts[1]);
}
}
return -1;
}
```
2. 调用Windows API获取窗口标题。可以使用Java的JNI技术调用Windows API获取指定进程的主窗口句柄,然后调用GetWindowText API获取窗口标题,具体实现可参考以下C++代码:
```c++
#include <windows.h>
#include <jni.h>
JNIEXPORT jstring JNICALL Java_WindowTitleGetter_getWindowTitle(JNIEnv *env, jclass cls, jint pid) {
HWND hwnd = NULL;
char title[1024];
// 获取指定进程的窗口句柄
hwnd = GetTopWindow(NULL);
while (hwnd != NULL) {
DWORD processId;
GetWindowThreadProcessId(hwnd, &processId);
if (processId == pid) {
DWORD style = GetWindowLong(hwnd, GWL_STYLE);
if ((style & WS_VISIBLE) != 0) {
break;
}
}
hwnd = GetNextWindow(hwnd, GW_HWNDNEXT);
}
// 获取窗口标题
if (hwnd != NULL) {
GetWindowTextA(hwnd, title, sizeof(title));
return env->NewStringUTF(title);
} else {
return env->NewStringUTF("");
}
}
```
3. 在Java中调用getWindowTitle方法即可获取指定应用的窗口标题,例如:
```java
String processName = "notepad.exe";
int pid = getProcessIdByName(processName);
String title = WindowTitleGetter.getWindowTitle(pid);
System.out.println(title);
```
注意:该方法需要使用管理员权限运行程序。
阅读全文
相关推荐



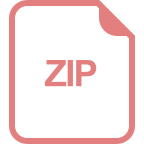
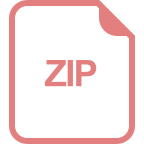
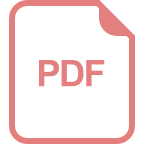
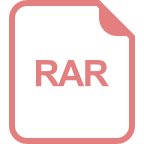
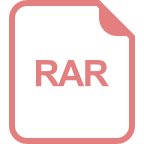
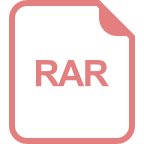
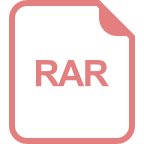
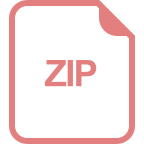
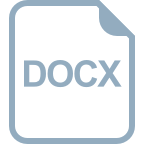
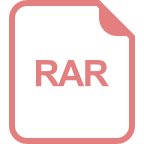
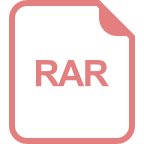
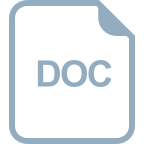
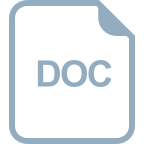