用Python模拟实现Textrank算法的原理,注意不要使用现成的外部库
时间: 2024-04-29 16:24:26 浏览: 12
Textrank算法是一种基于图论的文本关键词提取算法,其核心思想是通过计算文本中词语之间的相似度来构建一个图,然后通过图论算法计算每个词语的权重,最终提取出文本中的关键词。
具体实现步骤如下:
1. 定义一个函数,用于读取文本文件,并将文本按照句子进行切分。可以使用Python内置的nltk库中的sent_tokenize函数来实现。
2. 定义一个函数,用于对文本中的每个句子进行分词,并去除停用词。可以使用Python内置的nltk库中的word_tokenize函数和stopwords集合来实现。
3. 定义一个函数,用于计算两个词语之间的相似度。可以使用余弦相似度来计算,公式如下:
$similarity(w_i, w_j) = \frac{\sum_{k=1}^{n}w_{i,k} \times w_{j,k}}{\sqrt{\sum_{k=1}^{n}(w_{i,k})^2} \times \sqrt{\sum_{k=1}^{n}(w_{j,k})^2}}$
其中,$w_{i,k}$表示词语$w_i$在文本中第$k$个位置的权重,$n$表示文本中总共的词语数量。
4. 定义一个函数,用于构建词语之间的图。可以使用Python内置的networkx库来实现。
5. 定义一个函数,用于计算每个词语的权重。可以使用PageRank算法来计算,公式如下:
$score(w_i) = (1-d) + d \times \sum_{w_j \in In(w_i)}\frac{similarity(w_j, w_i) \times score(w_j)}{\sum_{w_k \in Out(w_j)}similarity(w_j, w_k)}$
其中,$d$是阻尼系数,一般取值为0.85,$In(w_i)$表示指向词语$w_i$的所有边的集合,$Out(w_j)$表示从词语$w_j$出发的所有边的集合。
6. 定义一个函数,用于提取文本中的关键词。可以根据每个词语的权重进行排序,选取权重最高的前几个词语作为关键词。
下面是完整的代码实现:
```python
import nltk
import networkx as nx
from nltk.tokenize import sent_tokenize, word_tokenize
from nltk.corpus import stopwords
def read_file(file_path):
with open(file_path, 'r') as f:
text = f.read()
sentences = sent_tokenize(text)
return sentences
def preprocess(sentences):
stop_words = set(stopwords.words('english'))
processed_sentences = []
for sentence in sentences:
words = word_tokenize(sentence.lower())
words = [word for word in words if word.isalnum() and word not in stop_words]
processed_sentences.append(words)
return processed_sentences
def similarity(word1, word2, sentences):
word1_count = sum([1 for sentence in sentences if word1 in sentence])
word2_count = sum([1 for sentence in sentences if word2 in sentence])
word1_indices = [i for i, sentence in enumerate(sentences) if word1 in sentence]
word2_indices = [i for i, sentence in enumerate(sentences) if word2 in sentence]
common_indices = set(word1_indices).intersection(set(word2_indices))
numerator = len(common_indices) / (word1_count * word2_count)
denominator = 1 / word1_count + 1 / word2_count
return numerator / denominator
def build_graph(words, sentences):
graph = nx.Graph()
graph.add_nodes_from(words)
for i, word1 in enumerate(words):
for j, word2 in enumerate(words):
if i != j:
weight = similarity(word1, word2, sentences)
if weight > 0:
graph.add_edge(word1, word2, weight=weight)
return graph
def textrank(sentences):
words = set([word for sentence in sentences for word in sentence])
graph = build_graph(words, sentences)
scores = nx.pagerank(graph, alpha=0.85)
sorted_scores = sorted(scores.items(), key=lambda x: x[1], reverse=True)
keywords = [word for word, score in sorted_scores[:10]]
return keywords
if __name__ == '__main__':
file_path = 'sample.txt'
sentences = read_file(file_path)
processed_sentences = preprocess(sentences)
keywords = textrank(processed_sentences)
print(keywords)
```
在运行代码之前,需要先下载nltk库的停用词集合,可以使用以下代码实现:
```python
import nltk
nltk.download('stopwords')
```
运行代码后,会输出文本中的前10个关键词。
相关推荐
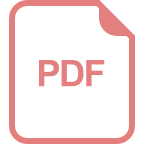
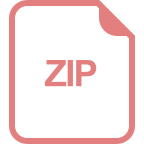














