帮我用c语言写一个每次摇一颗骰子的函数
时间: 2023-06-10 18:04:25 浏览: 111
好的,以下是用 C 语言编写的每次摇一颗骰子的函数:
```c
#include <stdlib.h> // For rand() and srand()
#include <time.h> // For time()
int rollDice() {
srand(time(NULL)); // Seed the random number generator with the current time
return rand() % 6 + 1; // Generate a random number between 1 and 6
}
```
使用方法:
```c
int main() {
int result = rollDice(); // Roll the dice
printf("The result is: %d", result); // Output the result
return 0;
}
```
此函数的功能是每次调用生成一个 1 到 6 的随机整数,模拟摇一颗骰子的结果。注意:为了让每次生成的结果都不同,需要在每次调用函数前使用 `srand()` 函数来设置随机数生成器的种子,可以通过传递 `time(NULL)` 函数的返回值来实现。
相关问题
帮我用c语言写一个摇骰子并每次累加点数的函数
好的,以下是用C语言实现的摇骰子并累加点数的函数:
```c
#include <stdlib.h>
#include <time.h>
int rollDice() {
int dice1, dice2, sum;
srand(time(NULL)); // 初始化随机数种子
dice1 = rand() % 6 + 1; // 掷第一颗骰子,随机数范围为1-6
dice2 = rand() % 6 + 1; // 掷第二颗骰子,随机数范围为1-6
sum = dice1 + dice2; // 求出两颗骰子点数的和
return sum; // 返回点数和
}
```
可以将上面的代码放到你的C程序中,通过调用 `rollDice` 函数来实现摇骰子并累加点数的功能。每次调用该函数,都会生成两个随机数模拟投掷两颗骰子,并计算出点数的总和。如果需要累加点数,可以在主函数里定义一个变量,每次调用 `rollDice` 函数时,累加点数和即可。
帮我用c语言写一个从确认开始到摇骰子的函数
好的,以下是一个简单的从确认开始到摇骰子的函数的C语言示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
int roll_dice(void); // 摇骰子函数
int main(void)
{
char confirmation;
int dice_value;
// 确认是否开始摇骰子
printf("Are you ready to roll the dice? (y/n) ");
scanf(" %c", &confirmation);
if (confirmation == 'y')
{
// 设置随机数种子
srand((unsigned) time(NULL));
// 开始摇骰子
dice_value = roll_dice();
printf("You rolled a %d!\n", dice_value);
}
else
{
printf("Maybe next time then.\n");
}
return 0;
}
// 摇骰子函数,返回骰子点数
int roll_dice(void)
{
int die1, die2;
die1 = rand() % 6 + 1;
die2 = rand() % 6 + 1;
return die1 + die2;
}
```
该程序首先询问用户是否准备好开始摇骰子。如果肯定回答,则设置随机数种子,调用摇骰子函数来生成一个随机数并输出,否则程序结束。
阅读全文
相关推荐
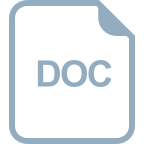
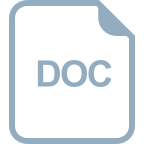
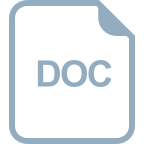
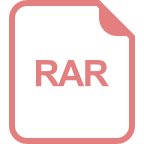
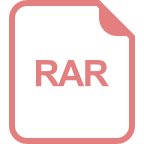
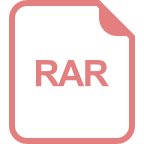
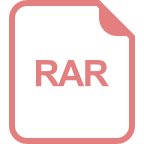
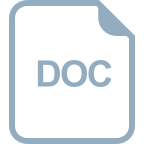
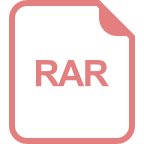
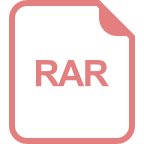
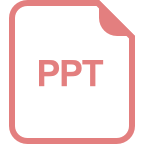
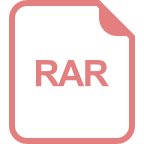
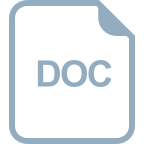