android studio设计一个音乐播放器
时间: 2023-11-05 20:57:44 浏览: 386
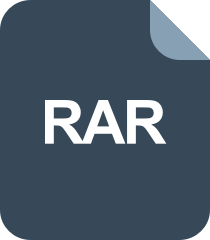
Android 音乐播放器设计
根据提供的引用内容,Android Studio可以用来设计一个音乐播放器。该项目涉及到Android基础知识、UI界面、数据存储、四大组件、网络编程和高级编程等技术。你可以综合运用UI界面设计、数据存储、Activity、Service、MusicPlayer、ListView等知识来完成这个音乐播放器的开发。你可以下载源码并参考博主提供的详细步骤和注释来帮助你完成这个项目。这样的练习对于以后的Android开发会有很大的帮助。
阅读全文
相关推荐
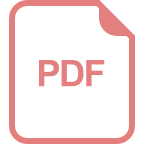


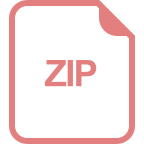
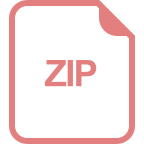





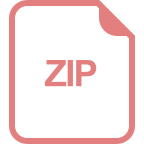
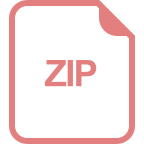
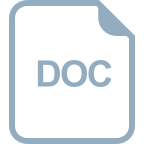
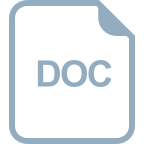


