java序列化与反序列化
时间: 2023-07-18 19:06:18 浏览: 115
Java序列化是将一个对象转换为字节序列的过程,以便在网络上传输或保存到本地文件系统中。Java反序列化则是将字节序列还原为原始的Java对象的过程。这个过程可以用于持久化对象状态,或者在分布式系统中进行对象的远程传输。
下面是一个简单的Java序列化和反序列化的例子:
```java
import java.io.*;
public class SerializationExample implements Serializable {
private String name;
private int age;
public SerializationExample(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
public static void main(String[] args) {
SerializationExample obj = new SerializationExample("John", 30);
try {
FileOutputStream fileOut = new FileOutputStream("example.ser");
ObjectOutputStream out = new ObjectOutputStream(fileOut);
out.writeObject(obj);
out.close();
fileOut.close();
System.out.println("Object serialized successfully.");
} catch (IOException e) {
e.printStackTrace();
}
try {
FileInputStream fileIn = new FileInputStream("example.ser");
ObjectInputStream in = new ObjectInputStream(fileIn);
SerializationExample newObj = (SerializationExample) in.readObject();
in.close();
fileIn.close();
System.out.println("Object deserialized successfully.");
System.out.println("Name: " + newObj.getName());
System.out.println("Age: " + newObj.getAge());
} catch (IOException | ClassNotFoundException e) {
e.printStackTrace();
}
}
}
```
在这个例子中,我们定义了一个`SerializationExample`类,并实现了`Serializable`接口。我们创建了一个对象`obj`,并将其序列化到文件中。然后,我们从文件中读取字节序列,并将其反序列化为一个新的对象`newObj`,并打印出其名称和年龄。
需要注意的是,如果想要序列化一个对象,该对象的所有非静态成员变量都必须是可序列化的。如果有一个非可序列化的成员变量,则会抛出`NotSerializableException`异常。
阅读全文
相关推荐
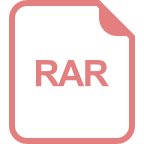
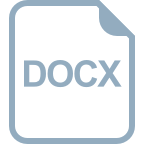
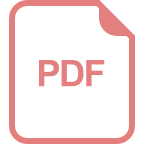
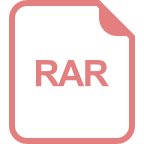
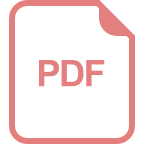
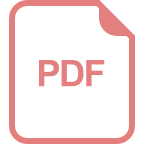
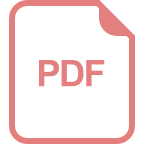
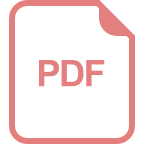
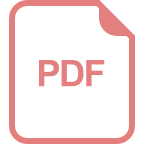
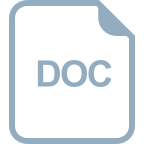
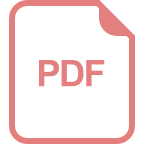
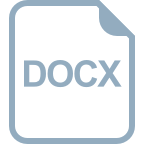
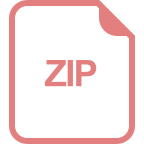
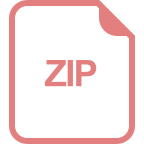