composition在java中的含义
时间: 2023-06-20 20:09:49 浏览: 53
在 Java 中,composition 是一种面向对象编程的概念,指一个类包含其他类作为其成员变量的一种关系。这种关系是一种 has-a 的关系,意味着一个对象包含其他对象作为其部分。与继承不同,组合关系中的成员变量通常是其他类的实例,而不是其他类的子类。
例如,如果我们有一个 Car 类和一个 Engine 类,Car 类需要一个 Engine 对象来驱动它。我们可以使用组合来实现这个关系,将 Engine 对象作为 Car 类的成员变量:
```
public class Engine {
// Engine 类的定义
}
public class Car {
private Engine engine;
public Car() {
engine = new Engine();
}
// Car 类的其他方法
}
```
在这个例子中,Car 类包含一个 Engine 对象作为其成员变量,我们可以通过 Car 对象来访问 Engine 对象的属性和方法。这种方式可以使我们更好地组织代码,提高代码的可读性和可维护性。
相关问题
java中composition
和inheritance有什么区别?
Composition和Inheritance是面向对象编程中的两种关系,都涉及到类之间的相互关系。
Composition是一个类包含另一个类的实例作为其成员变量或属性,通过包含其他类的实例来实现功能。这种关系通常是“有一个”的关系,例如汽车包含引擎。
Inheritance是一个类继承另一个类,它获得了其父类的所有方法和属性。这种关系通常是“是一个”的关系,例如狗是动物的一种。
因此,Composition强调组合、聚合、依赖等关系,而Inheritance强调类之间的继承关系。
java composition
Java composition is a design technique in object-oriented programming (OOP) where objects are combined to form more complex objects or systems. Composition is a way to create relationships between classes that allow them to work together to achieve a common goal.
In Java, composition is achieved by creating objects of other classes within a class. These objects become part of the class and can be accessed by its methods. The class that contains the objects is called the composite class, while the objects that are part of it are called component classes.
For example, imagine a Car class that has a Headlight class and an Engine class as its components. The Car class can use the Headlight and Engine objects to perform its functions, such as turning on the headlights or starting the engine. The Headlight and Engine classes can also be used independently of the Car class, making them reusable and modular.
Composition provides several benefits in Java programming, including:
1. Flexibility: Composition allows for more flexibility in designing classes and systems. Components can be easily added or removed from the composite class without affecting other parts of the system.
2. Code reuse: Components can be used in multiple composite classes, reducing code duplication and improving maintainability.
3. Encapsulation: Components are encapsulated within the composite class, preventing direct access from outside the class and improving data security.
4. Polymorphism: Components can be replaced with other objects that have the same interface, allowing for polymorphic behavior.
In summary, Java composition is a powerful design technique that enables developers to create complex systems by combining simple objects. It provides flexibility, code reuse, encapsulation, and polymorphism, making it a valuable tool in object-oriented programming.
相关推荐
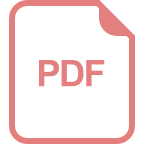
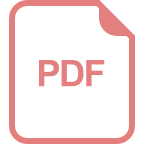
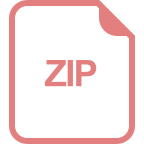












