linux c 指针型指针
时间: 2025-01-03 12:35:04 浏览: 8
### C语言 Linux 环境下指针使用教程
#### 定义和初始化指针变量
在C语言中,指针是一种特殊的变量,用于存储另一个变量的内存地址。定义一个指针时需指定其指向的数据类型。
```c
#include <stdio.h>
int main() {
int a = 68;
int *p = &a;
printf("Value of 'a': %d\n", a);
printf("Address of 'a' (&a): %p\n", (void*)&a);
printf("Value stored in pointer 'p': %p\n", (void*)p);
printf("Dereferenced value through 'p' (*p): %d\n", *p);
return 0;
}
```
上述程序展示了如何声明整数类型的指针`p`并将其初始化为指向变量`a`的地址[^2]。通过打印输出可以看到,`*p`能够访问到`a`的实际数值。
#### 函数中的指针参数传递
当向函数传入指针作为参数时,可以在不改变原值的情况下修改调用者空间内的数据对象。下面的例子演示了两个整数交换的过程:
```c
void swap(int *xp, int *yp) {
int temp = *xp;
*xp = *yp;
*yp = temp;
}
int main() {
int x = 10, y = 20;
printf("Before swapping: x = %d, y = %d\n", x, y);
swap(&x, &y);
printf("After swapping: x = %d, y = %d\n", x, y);
return 0;
}
```
这段代码实现了简单的两数互换操作,其中`swap()`接受一对指向整型的指针来实现这一功能[^1]。
#### 结合多线程环境下的指针运用
对于Linux平台上的并发编程而言,利用POSIX Threads(Pthreads),可以方便地创建新线程执行特定任务。这里给出一个多线程示例,在主线程之外启动子线程计算斐波那契序列的部分项,并借助指针完成结果回传给父进程的工作。
```c
#include <pthread.h>
#include <stdio.h>
// 子线程工作负载:计算第n个斐波那契数列成员
void *fibonacci(void *param) {
long n = *(long *)param;
if(n <= 1){
pthread_exit((void *)(intptr_t)n);
}
long prev = 0, curr = 1;
for(long i = 2; i <= n ; ++i){
long next = prev + curr;
prev = curr;
curr = next;
}
pthread_exit((void *)(intptr_t)curr);
}
int main(){
pthread_t tid;
long num = 7L; // 计算Fib(7)
/* 启动新的线程 */
pthread_create(&tid, NULL, fibonacci, (void *)&num);
/* 获取子线程返回的结果 */
void *result;
pthread_join(tid, &result);
printf("The Fibonacci number F(%ld) is %ld.\n", num, (long)(uintptr_t)result);
return 0;
}
```
此段源码片段里包含了对`pthread_create()` API 的调用以及相应回调函数的设计;值得注意的是,为了简化起见,这里采用`(void*)(intptr_t)`的方式来进行基本数据类型的转换处理[^4]。
阅读全文
相关推荐



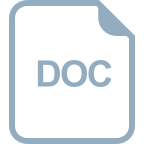













