java抽象工厂模式简单代码
时间: 2023-12-04 18:41:33 浏览: 99
抽象工厂模式是一种创建型设计模式,它允许客户端通过接口创建一组相关的对象,而无需指定其具体类。
以下是一个简单的 Java 抽象工厂模式的代码示例:
```
// 抽象工厂接口
interface AbstractFactory {
public Shape createShape();
public Color createColor();
}
// 具体工厂实现类1
class ConcreteFactory1 implements AbstractFactory {
public Shape createShape() {
return new Circle();
}
public Color createColor() {
return new Red();
}
}
// 具体工厂实现类2
class ConcreteFactory2 implements AbstractFactory {
public Shape createShape() {
return new Square();
}
public Color createColor() {
return new Blue(); }
}
// 抽象产品接口1
interface Shape {
public void draw();
}
// 具体产品实现类1
class Circle implements Shape {
public void draw() {
System.out.println("Inside Circle::draw() method.");
}
}
// 具体产品实现类2
class Square implements Shape {
public void draw() {
System.out.println("Inside Square::draw() method.");
}
}
// 抽象产品接口2
interface Color {
public void fill();
}
// 具体产品实现类3
class Red implements Color {
public void fill() {
System.out.println("Inside Red::fill() method.");
}
}
// 具体产品实现类4
class Blue implements Color {
public void fill() {
System.out.println("Inside Blue::fill() method.");
}
}
// 客户端代码
public class Client {
public static void main(String[] args) {
AbstractFactory factory1 = new ConcreteFactory1();
Shape shape1 = factory1.createShape();
Color color1 = factory1.createColor();
shape1.draw();
color1.fill();
AbstractFactory factory2 = new ConcreteFactory2();
Shape shape2 = factory2.createShape();
Color color2 = factory2.createColor();
shape2.draw();
color2.fill();
}
}
```
在上面的代码中,`AbstractFactory` 是抽象工厂接口,定义了创建产品的方法。`ConcreteFactory1` 和 `ConcreteFactory2` 是具体工厂实现类,分别创建 `Circle` 和 `Red` 或者 `Square` 和 `Blue` 两个相关的产品。`Shape` 和 `Color` 是抽象产品接口,定义了产品的方法。`Circle`、`Square`、`Red` 和 `Blue` 是具体产品实现类,实现了抽象产品接口中定义的方法。
在客户端代码中,我们可以通过具体工厂实现类来创建一组相关的产品,并调用其方法。
--相关问题--:
1. 抽象工厂模式是什么?
2. 抽象工厂模式有哪些角色
阅读全文
相关推荐





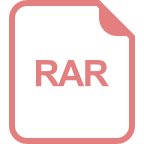












