用c语言在Linux操作系统中,实现万年历,并提供友好的用户界面
时间: 2023-07-19 17:29:38 浏览: 146
好的,以下是用C语言实现Linux操作系统中万年历的代码,代码中使用了ncurses库来提供友好的用户界面:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <time.h>
#include <ncurses.h>
#define MAX_CAL_ROW 9 // 日历的最大行数
#define MAX_CAL_COL 21 // 日历的最大列数
// 获取某一年某一月的天数
int get_month_days(int year, int month)
{
if (month == 2)
{
if (year % 4 == 0 && year % 100 != 0 || year % 400 == 0)
{
return 29;
}
else
{
return 28;
}
}
else if (month == 4 || month == 6 || month == 9 || month == 11)
{
return 30;
}
else
{
return 31;
}
}
// 获取某一年某一月的第一天是星期几(0表示星期天,1表示星期一,以此类推)
int get_month_first_day(int year, int month)
{
char date_str[20];
snprintf(date_str, sizeof(date_str), "%d-%d-1", year, month);
struct tm time_struct;
strptime(date_str, "%Y-%m-%d", &time_struct);
time_t time_stamp = mktime(&time_struct);
return localtime(&time_stamp)->tm_wday;
}
// 绘制日历
void draw_calendar(int year, int month)
{
// 清空屏幕
clear();
// 设置窗口大小
int row, col;
getmaxyx(stdscr, row, col);
int cal_row = MAX_CAL_ROW;
int cal_col = MAX_CAL_COL;
if (row < cal_row || col < cal_col)
{
endwin();
printf("窗口太小,无法显示日历!\n");
exit(EXIT_FAILURE);
}
// 绘制日历
char month_str[30];
snprintf(month_str, sizeof(month_str), "%d年%d月", year, month);
mvaddstr(0, (col - strlen(month_str)) / 2, month_str);
mvaddstr(2, 0, "日 一 二 三 四 五 六");
int month_days = get_month_days(year, month);
int month_first_day = get_month_first_day(year, month);
int row_index = 3;
int col_index = month_first_day * 3;
for (int i = 1; i <= month_days; i++)
{
char day_str[3];
snprintf(day_str, sizeof(day_str), "%d", i);
mvaddstr(row_index, col_index, day_str);
if (col_index == 6 * 3)
{
row_index++;
col_index = 0;
}
else
{
col_index += 3;
}
}
refresh();
}
int main()
{
// 初始化ncurses库
initscr();
// 设置ncurses库参数
cbreak();
noecho();
keypad(stdscr, TRUE);
// 获取当前日期
time_t now = time(NULL);
struct tm *now_tm = localtime(&now);
int year = now_tm->tm_year + 1900;
int month = now_tm->tm_mon + 1;
// 绘制初始日历
draw_calendar(year, month);
// 处理用户输入
int input;
while ((input = getch()) != 'q')
{
switch (input)
{
case KEY_LEFT:
if (month == 1)
{
year--;
month = 12;
}
else
{
month--;
}
draw_calendar(year, month);
break;
case KEY_RIGHT:
if (month == 12)
{
year++;
month = 1;
}
else
{
month++;
}
draw_calendar(year, month);
break;
default:
break;
}
}
// 结束ncurses库
endwin();
return 0;
}
```
以上代码通过ncurses库提供了一个友好的用户界面,用户可以使用箭头键左右切换月份,使用q键退出程序。通过计算每个月的天数和第一天是星期几,绘制了相应的日历。
阅读全文
相关推荐










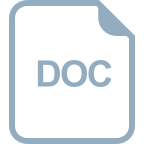
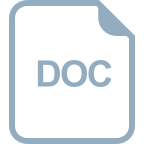







