import threading import time import sys class Countdown(threading.Thread): def __init__(self): super().__init__() self.stopped = False def run(self): for i in range(60, 0, -1): sys.stdout.write('\r' + '剩余时间:{:2d}秒'.format(i)) sys.stdout.flush() time.sleep(1) if self.stopped: break if not self.stopped: sys.stdout.write('\r' + '倒计时结束! \n') sys.stdout.flush() def stop(self): self.stopped = True if __name__ == '__main__': while True: countdown = Countdown() countdown.start() user_input = input('是否停止倒计时?(y/no)') countdown.stop() if user_input.lower() == 'y': break
时间: 2024-02-14 09:20:27 浏览: 112
这段代码是一个倒计时程序,它使用了 Python 的 threading 模块来实现多线程。在主线程中,每次循环都会创建一个新的 Countdown 线程,并启动它。同时,主线程会等待用户输入,如果用户输入 y,则会停止倒计时线程。
在 Countdown 线程中,run() 方法会循环60次,每次输出剩余时间,并通过 time.sleep(1) 来让线程休眠1秒。同时,如果 self.stopped 被设置为 True,则会跳出循环,结束线程。stop() 方法则用来设置 self.stopped 的值,从而停止线程。
需要注意的是,在每次循环中,使用了 sys.stdout.write() 来输出文字,并使用了 \r 来实现覆盖输出,这样可以让输出的文字在同一行上不断更新。对于 flush() 方法,则是用来强制将输出立即打印到屏幕上,而不是等到缓存区满了才打印。
相关问题
class myThread (threading.Thread): def __init__(self): threading.Thread.__init__(self)
这段代码定义了一个名为myThread的类,它继承自threading.Thread类。这个类中定义了一个构造函数__init__,在这个函数中调用了父类的构造函数来初始化线程。
具体来说,__init__函数中的代码是:
```python
def __init__(self):
threading.Thread.__init__(self)
```
这个代码调用了父类(threading.Thread)的构造函数,并将self作为参数传入。这样就可以初始化一个线程对象了。
在这个类中,还可以定义其他的方法,这些方法将在子线程中执行。在本例中,run方法是线程的主体,它会在子线程中被执行。因此,在定义myThread类时,需要重写run方法。
from queue import Queue import random, threading, time # 生产者类 class Producer(threading.Thread): def __init__(self, name, queue):
这是一个Python代码片段,定义了一个名为`Producer`的生产者线程类,它继承自`threading.Thread`,这意味着这个类可以创建一个新的线程来执行其任务。以下是类的主要部分:
1. `__init__`函数是一个构造函数,当创建`Producer`实例时自动调用。它接受两个参数:
- `name`: 通常用于识别线程,这里是线程的名称,如"Producer_1"。
- `queue`: 用于存储产品的一个`Queue`对象。`Queue`是Python标准库提供的线程安全队列,可以在多个线程之间共享数据。
生产者类的工作原理通常是这样的:
- 当创建一个`Producer`实例并开始运行(通过`start()`方法)时,该线程会进入循环,不断尝试将一些数据(“产品”)放入队列中。
- 数据生成和添加到队列的过程由`run`方法内部的逻辑控制,这部分未在这个代码片段中展示。
```python
class Producer(threading.Thread):
def __init__(self, name, queue):
super().__init__(name=name) # 调用父类的初始化方法
self.queue = queue
def run(self):
while True: # 这里应该有数据生成和添加队列的操作
product = generate_product() # 模拟生成产品的函数
self.queue.put(product)
time.sleep(random.randint(1, 5)) # 随机暂停一段时间
def generate_product(): # 未在此代码中定义,可能是生成数字、字符串或其他对象的函数
pass
# 创建生产者实例并启动
producer = Producer("Producer", Queue())
producer.start()
```
阅读全文
相关推荐
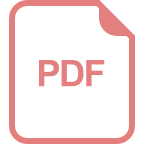
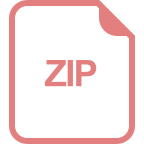
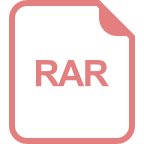














