javaGUI制作一个加法练习器
时间: 2023-11-11 15:06:51 浏览: 46
好的,以下是Java GUI制作一个加法练习器的代码示例:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class AdditionPractice extends JFrame implements ActionListener {
private JTextField num1Field, num2Field, answerField;
private JLabel feedbackLabel;
private JButton checkButton, newProblemButton;
public AdditionPractice() {
// 设置窗口标题
setTitle("加法练习器");
// 创建面板
JPanel panel = new JPanel();
panel.setLayout(new GridLayout(4, 2, 5, 5));
// 添加数字1输入框
panel.add(new JLabel("数字1:"));
num1Field = new JTextField(10);
panel.add(num1Field);
// 添加数字2输入框
panel.add(new JLabel("数字2:"));
num2Field = new JTextField(10);
panel.add(num2Field);
// 添加答案输入框
panel.add(new JLabel("答案:"));
answerField = new JTextField(10);
panel.add(answerField);
// 添加“检查”按钮
checkButton = new JButton("检查");
checkButton.addActionListener(this);
panel.add(checkButton);
// 添加“新问题”按钮
newProblemButton = new JButton("新问题");
newProblemButton.addActionListener(this);
panel.add(newProblemButton);
// 添加反馈标签
feedbackLabel = new JLabel("");
panel.add(feedbackLabel);
// 添加面板到窗口
add(panel);
// 设置窗口大小和可见性
setSize(300, 200);
setVisible(true);
}
// ActionListener接口方法
public void actionPerformed(ActionEvent e) {
if (e.getSource() == checkButton) {
// 检查答案
int num1 = Integer.parseInt(num1Field.getText());
int num2 = Integer.parseInt(num2Field.getText());
int answer = Integer.parseInt(answerField.getText());
if (answer == num1 + num2) {
feedbackLabel.setText("回答正确!");
} else {
feedbackLabel.setText("回答错误!");
}
} else if (e.getSource() == newProblemButton) {
// 生成新问题
num1Field.setText("" + (int)(Math.random() * 10));
num2Field.setText("" + (int)(Math.random() * 10));
answerField.setText("");
feedbackLabel.setText("");
}
}
public static void main(String[] args) {
new AdditionPractice();
}
}
```
这个加法练习器包括数字1、数字2和答案的输入框,一个“检查”按钮和一个“新问题”按钮,以及一个反馈标签。当用户点击“检查”按钮时,程序将检查答案是否正确。当用户点击“新问题”按钮时,程序将生成一个新的加法问题。
相关推荐
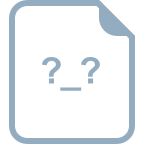
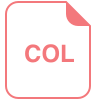
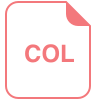
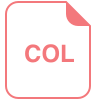
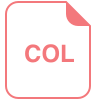
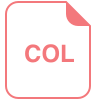









